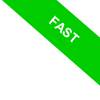
Mathematical Operations in Matlab
In this lesson, I'll explain how to perform mathematical calculations in Matlab and introduce the symbols for the primary mathematical operators.
Addition
The symbol for addition is the plus sign +
>> 3+2
ans = 5
Subtraction
The symbol for subtraction is the minus sign -
>> 3-2
ans = 1
Multiplication
The symbol for multiplication is the asterisk *
>> 4*2
ans = 8
Division
The symbol for division is the forward slash or slash /
>> 8/2
ans = 4
Remainder of Division
To calculate the remainder of division, use the mod() function.
>> mod(7,2)
ans = 1
Note. In Matlab, you can't use the % symbol to find the remainder of a division because it's already used to insert comments in the code. So if you write 7%2, Matlab will treat 2 as a comment. The only way to calculate the remainder of a division is by using the mod() function.
Exponentiation
The symbol for exponentiation is ^
>> 2^3
ans = 8
Square Root
To calculate the square root of a number, use the sqrt() function.
>> sqrt(16)
ans = 4
Alternatively, you can raise the number to 1/2.
>> 16**(1/2)
ans = 4
Other Mathematical Functions
Matlab has a large number of predefined functions to perform specific mathematical operations. Here are some examples:
- abs(x)
returns the absolute value of x - cos(x)
calculates the cosine of x - sin(x)
calculates the sine of x - tan(x)
calculates the tangent of x - acos(x)
calculates the arccosine of x - asin(x)
calculates the arcsine of x - atan(x)
- calculates the arctangent x
- exp(x)
calculates the exponential of x - log(x)
calculates the natural logarithm of x - log10(x)
calculates the base 10 logarithm of x - sqrt(x)
calculates the square root of x - nthroot(x,n)
calculates the real nth root of x
For example, if you want to calculate the absolute value of a number, use the abs() function.
>> abs(-1)
ans = 1
Note. In general, Matlab results are displayed in short format with 5 significant digits by default. However, you can change the format from short to long or use scientific notation.