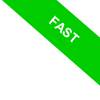
Computing the nth Root with Python
Welcome to this comprehensive guide on calculating the nth root in Python
First, let's clarify: what is the nth root? It's a value that, when raised to the power of 'n', returns our original number. For example, the cube root (where n=3) of 8 is 2, demonstrated by the equation 23 = 8. $$ \sqrt[3]{8} = 2 $$ Similarly, the fourth root (where n=4) of 16 is 2, given that 24 = 16. $$ \sqrt[4]{16} = 2 $$
Python offers an elegant and straightforward way to compute nth roots using the power function, pow(). Here's the formula:
pow(x, 1/n)
This method is rooted in a fundamental property of powers:
$$ \sqrt[n]{x} = x^{ \frac{1}{n} } $$
For a more tangible understanding, consider this scenario: you wish to find the cube root of 27.
$$ \sqrt[3]{27} $$
In Python, you can effortlessly achieve this with pow(27, 1/3)
pow(27,1/3)
The result? A neat 3, because 33 = 27, with the actual return value being 3.0.
3.0
Beyond the cube root, Python's versatility allows you to compute a plethora of other nth roots.
Let's explore the fourth root of 16.
$$ \sqrt[4]{16} $$
Execute the pow() function as follows:
pow(16,1/4)
The answer is 2, stemming from the equation 24 = 16, and it's rendered as 2.0.
2.0
For those keen on optimizing their code, crafting a dedicated function can be a savvy move.
def th_root(x, n):
return pow(x, 1/n)
Once established, call upon this function with your desired values. For the cube root of 27.
th_root(27,3)
The consistent outcome is 3.0.
3.0
Alternatively, you can use the Python's power operator.
27**(1/3)
Yet again, we're greeted with a result of 3.0.
3.0
For those looking to dive even deeper, the nth root can also be unearthed through the properties of logarithms and exponentials.
$$ \sqrt[n]{x} = e^{ \frac{ \log(x) }{n} } $$
To embark on this journey, incorporate the log() and exp() functions from Python's math module.
math.exp(math.log(x) / n)
For our ever-familiar cube root of 27.
math.exp(math.log(27) / 3)
And as has become expected, the result stands firm at 3.0.
3.0
While the pow(x, 1/n) function typically suffices for most applications, the versatility of Python allows developers an array of methods.
Equipping oneself with these various approaches can undoubtedly prove advantageous in nuanced scenarios