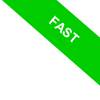
Numbers in Python
In the world of Python, numbers play a pivotal role as one of the core data types. Let's delve into the various categories and nuances of these numerical types.
Integers
Simply put, integers are whole numbers. They lack a decimal component, and they can range from negative to positive values, including zero. In Python's realm, we denote integers with the `int` type.
x = 5
type(x)
<class 'int'>
Python offers a versatile approach to representing integers. You can express them in decimal (base 10), binary (base 2), octal (base 8), or even hexadecimal (base 16) formats.
Floating-Point Numbers
Floating-point numbers introduce a decimal component. In Python, these numbers find their identity with the "float" type.
x = 5.7
type(x)
<class 'float'>
Booleans
Booleans are the embodiment of binary logic in programming: true or false. In Python, this duality is captured by the "bool" type.
x =False
type(x)
<class 'bool'>
Fractions
For those who need to work with exact fractional values, Python's fractions module is a godsend.
from fractions import Fraction
x = Fraction(3, 4)
type(x)
<class 'fractions.Fraction'>
Decimals
For those who seek precision, especially in financial computations, the decimal module offers decimal numbers with customizable precision.
from decimal import Decimal
x = Decimal('0.1')
type(x)
<class 'decimal.Decimal'>
Complex Numbers
Complex numbers are a blend of real and imaginary components. Python elegantly represents them using the "complex" type.
x = 3 + 4j
type(x)
<class 'complex'>
Type Conversions
Python facilitates seamless transitions between numeric types using built-in functions such as int(), float(), and complex().
The numbers.Number Type
In Python, every number, regardless of its type, can be viewed as an immutable instance of the broader "numbers.Number" category.
For instance, the number 5 is an instance of the int type:
isinstance(5, int)
True
On the other hand, the number 5 is also an instance of the numbers.Number type:
import numbers
print(isinstance(5, numbers.Number))
True
The same holds true for all other numbers and numeric types.