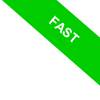
Fractions in Python
Python provides an intuitive way to represent and manipulate fractions (rational numbers) through its fractions module.
import fractions
By leveraging this module, you can harness the power of the Fraction class: <class 'fractions.Fraction'>
This class facilitates the creation of fraction objects and seamlessly handles all arithmetic operations between them.
For those seeking precision in their calculations, such as in symbolic or financial mathematics, the fractions module is indispensable.
Let's dive into a practical example.
To get started, simply import the `Fraction` class from the `fractions` module:
from fractions import Fraction
It's worth noting that a module only needs to be imported once at the outset of your script or session.
Upon importing the `Fraction` class, you can instantiate a fraction using the Fraction(n,d) constructor
Fraction(n,d)
This constructor expects two parameters: the numerator "n" and the denominator "d".
For instance, to represent the fraction 3/4 it in the variable "f1", you'd use Fraction(3, 4)
f1 = Fraction(3, 4)
Alternatively, the fraction can be instantiated using a string format '3/4'
f1 = Fraction('3/4')
or even a floating-point representation (0.75)
f1 = Fraction(0.75)
To ascertain the data type of your variable, employ the type() function:
type(f1)
Python will affirmatively indicate that "f1" is an instance of the fractions.Fraction class
<class 'fractions.Fraction'>
Let's proceed by creating another fraction, 7/2, and store it in the variable "f2".
f2 = Fraction(7, 2)
With these fractions at your disposal, you're equipped to perform a full suite of arithmetic operations.
For example, to add the two fractions:
f1+f2
17/4
To subtract the two fractions:
f1-f2
-5/4
You can also multiply the fractions:
f1*f2
21/8
And divide the fractions:
f1/f2
3/14
In all cases, the result is always a fraction in their simplest form.
Wondering how to access the numerator and denominator directly?
To access the numerator and denominator of a fraction, you can use the `numerator` and `denominator` properties of the objects.
For instance, to get the numerator of the fraction, use f1.numerator
f1.numerator
Python will return the numerator of the fraction f1=3/4, which is 3
3
To access the denominator, use f1.denominator
f1.denominator
Python returns the denominator of the fraction f1=3/4 which is 4
4
And how about converting fractions to a rational number?
Should you need to convert a fraction to a decimal representation, the float function is at your service.
For example, type float(f1) to convert the "f1" variable
float(f1)
Given that "f1" is assigned the fraction 3/4, the floating-point value of the fraction is the result of the division 3/4, which is 0.75.
0.75
With this knowledge in hand, you're primed to harness the full potential of fractions in Python.