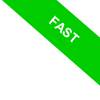
The Infinity Symbol in Python
Python doesn't have a dedicated symbol for infinity (∞). But don't fret! You can easily represent positive infinity (+∞) using the float() function paired with the special string "inf" or 'infinity'.
float('inf')
For negative infinity (-∞), it's just as straightforward:
float('-inf')
Python recognizes these values as the mathematical concept of infinity.
When displaying operation results, Python opts for the "inf" notation. However, this notation isn't for direct variable assignment. To explicitly set a variable to infinity, employ the float() function with either 'inf' or 'infinity' enclosed in parentheses.
Let's dive into an example.
Set the variable "x" to the special value float('inf')
x = float('inf')
Now, unveil the content of "x"
print(x)
You'll be greeted with the "inf" notation.
inf
In mathematical operations, Python embraces the float('inf') value as its representation of infinity.
Curious about adding 1 to infinity? Give this a whirl:
print(float('inf')+1)
Unsurprisingly, the sum remains "inf"
inf
How about a comparison between positive infinity and a mere mortal number?
print(1000 < float('inf'))
As expected, Python confirms with a resounding True.
True
But tread carefully. Infinity can be a tricky beast. Not every operation involving it produces intuitive outcomes.
When faced with undefined mathematical operations, Python resorts to the nan symbol (Not a Number).
Consider dividing positive infinity by its negative counterpart:
x = float('inf')
y = float('-inf')
print(x/y)
The conundrum of ∞/∞ leaves Python with no choice but to return nan
nan
This flexibility ensures that infinity can be wielded in calculations and comparisons just like any conventional number.