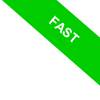
Rounding Numbers in Python
In today's tutorial, we'll explore the nuances of rounding numbers in Python. Python offers an array of functions and methods for this purpose, catering to diverse needs.
The round() Function
The round() function is the go-to for many when it comes to rounding. It provides precision, allowing you to specify the number of decimal places.
round(n,d)
Where `n` represents the number you wish to round, and `d` dictates the number of decimal places.
Omitting the second argument defaults the function to round to the nearest whole number.
For clarity, rounding the number 3.5678 to two decimal places would look like:
round(3.5678,2)
This returns 3.57 - the closest two-decimal representation.
3.57
But, what if you drop the second argument?
round(3.5678)
In this scenario, the function yields the nearest integer, which is 4.
4
The int() Function
If truncation is what you're after, the int() function is your ally.
It efficiently discards the decimal portion, delivering a neat integer.
int(n)
Here's a hands-on example:
int(3.5678)
This gives a straight 3. The decimal tail? Gone.
3
Give it a shot with a negative number:
int(-3.5678)
Even in this situation, the function simply removes the decimal digits, giving -3.
-3
The math.floor() Function
Enter the math.floor() function. Part of the math module, this function rounds numbers down to the closest integer.
Remember to import the module before diving in.
import math
Using our recurring example, rounding 3.5678 with math.floor()
math.floor(3.5678)
Hands you a 3, the nearest lower integer.
3
And for negative values?
math.floor(-3.5678)
For this, the closest lower integer is -4
-4
The math.ceil() Function
The `math.ceil()` function, another gem from the math module, serves the opposite purpose.
It rounds numbers up, always aiming high. As always, an import is essential.
import math
Rounding 3.5678 using math.ceil()
math.ceil(3.5678)
The nearest higher integer is 4.
4
For a twist, use a negative value:
math.ceil(-3.5678)
In this instance, the nearest higher integer is -3.
-3
Rounding with String Formatting
String formatting can be a handy tool for rounding, especially when you're looking to display results with precision.
To round 3.5678 to two decimal places:
print(f"{3.5678:.2f}")
Python gracefully rounds this to 3.57.
3.57
In conclusion, Python equips you with a robust set of tools for rounding numbers. Whether you're seeking precision, truncation, or formatting elegance, Python has you covered.