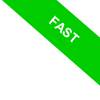
Mathematical Operations in Python
In this lesson, I will explain how to perform the main mathematical operations in the Python language.
Addition
The addition operator is the symbol "+"
>>> 1+2
3
Subtraction
The subtraction operator is the symbol "-"
>>> 4-1
3
Multiplication
The multiplication operator is the symbol "*"
>>> 3*4
12
Division
The division operator is the symbol "/"
>>> 10/4
2.5
The result of the division is always a float.
For example, the result of the division between the two integers 12/4 is 3.0
>>12/4
3.0
The result is a float type.
>>> type(12/4)
<class 'float'>
Floor Division
The floor division operator is the symbol "//".
Floor division rounds the quotient of the division down to the nearest integer.
>>> 10//4
2
In this case, the result is always an integer type.
>>> type(10//4)
<class 'int'>
Modulo
The modulo operation gives the remainder of a floor (integer) division. It's represented by the % symbol.
For instance, when dividing 10 by 3, the remainder is 1, as 3 times 3 equals 9.
>>> 10%3
1
It's worth noting that the result is always an integer type (int).
Exponentiation
The exponentiation operator is the symbol "**"
>>> 2**4
16
I hope this lesson helps you understand how to perform basic mathematical operations in Python.