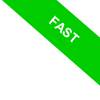
Binary Numbers in Python
Welcome to this tutorial on binary numbers in Python. If you're looking to understand how integers are represented in binary using Python, you're in the right place.
Understanding Binary Numbers. Binary numbers are fundamental to data representation in the world of computing. Unlike our familiar decimal system which uses digits from 0 to 9, binary numbers rely solely on two digits: 0 and 1. This is the essence of base-2 arithmetic. Python, being the versatile language that it is, offers a suite of powerful tools to seamlessly work with these numbers, facilitating easy conversions, arithmetic, and bit-level operations.
Assigning Binary Values in Python
To denote a binary value in Python, simply prefix your number with "0b" or "0B".
For instance, the decimal number 7, represented in binary as "111", can be written as:
x=0b111
And yes, the "b" is case-insensitive.
x=0B111
The final outcome is the binary representation of the decimal number 7, which is 111.
The value returned starts with '0b', signifying it's in binary format.
print(x)
0b111
To elegantly display numbers in binary, Python's string formatting comes to the rescue. You can also use string formatting with the "b" symbol:
num = 7
print(f"{num:b}")
This will yield the binary number 111.
111
Alternatively, you can use the bin() function which converts a decimal value into a binary number with the '0b' prefix.
num=bin(7)
This will give you '0b111'.
'0b111'
Keep in mind that the bin() function returns a string representation of the binary number, not the actual binary number itself. So, if you want to use the result in a mathematical operation, you'll need to convert it first.
int(num,2)
In doing so, you get the equivalent whole number, which is 7.
7
For those times when you need to revert from binary to decimal, the int() function is your ally
y=int(0b111)
This translates the binary number 111 back to its decimal form, 7.
print(y)
7
Python doesn't shy away from arithmetic operations on binary numbers.
Adding, subtracting, multiplying, or dividing these numbers is as straightforward as with decimals.
However, remember that the result will be in decimal form by default. To retrieve the binary result, simply wrap the operation with the bin() function.
Let's illustrate with two binary numbers: `1010` (10 in decimal) and `101` (5 in decimal):
a = 0b1010
b = 0b0101
You can add the two binary numbers using the + operator:
a+b
15
Note that Python returns the operation's result in decimal representation (15).
If you want the result in binary, you'd convert it using the bin() function.
bin(a+b)
0b1111
You can subtract the two binary numbers using the - operator:
a-b
5
Multiply the two binary numbers using the * operator:
a*b
50
Divide the two binary numbers:
a/b
2.0
Bit-Level Operations
Python also offers a rich set of operators for bit-level manipulations:
- Bitwise AND (&)
Returns a number where a bit is set if it was set in both numbers. The Bitwise AND operator in Python performs a logical AND operation on each pair of corresponding bits of the two numbers, returning a new binary number where a bit is set to 1 only if both corresponding bits in the original numbers are 1.
bin(a & b)
0b0
- Bitwise OR (|)
Sets a bit if it was set in either number. The Bitwise OR operator combines the bits of two binary numbers so that the resulting bit in each position is 1 if at least one of the corresponding bits in the original numbers is 1, otherwise it's 0.
bin(a | b)
0b1111
- Bitwise XOR (^)
Sets a bit if it was set in one number but not both. The Bitwise XOR (exclusive OR) operator compares two numbers bit by bit, returning a binary number where a bit is set to 1 if and only if one of the two corresponding bits in the original numbers is 1, but not both.
bin(a ^ b)
0b1111
- Bitwise NOT (~)
Inverts all the bits. The Bitwise NOT operator inverts all bits of a number, turning every 0 bit into a 1 and every 1 bit into a 0.
bin(~a)
-0b1011
- Left Shift (<<)
Shifts the bits of a number to the left. The Left Shift is a binary operation that moves all bits of a number to the left by a specified number of positions, filling the resulting empty bits with zeros. For instance, type a<<1 to shift by just one bit to the left:
bin(a << 1)
0b10100
- Right Shift (>>)
Shifts the bits of a number to the right. The Right Shift is a binary operation that moves all bits of a number to the right by a specified number of positions, filling the resulting empty bits with zeros. For instance, type a>>1 to shift by just one bit to the right:
bin(a >> 1)
0b101
Armed with this knowledge, you're now well-equipped to navigate the world of binary numbers in Python. Whether you're performing basic arithmetic or diving deep into bit-level operations, Python has got you covered.