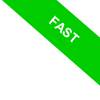
Sets in Python
In this lesson, I will explain how to create a set and perform basic operations on sets in the Python programming language.
What is a set? In mathematics, a set is a collection of objects known as "elements" of the set. The typical characteristics of a set are:
- A set can simultaneously contain elements of different types. For example, numbers and letters.
- Each element can only appear once in the set. Therefore, there can be no duplicate elements in a set.
- The elements in the set are not ordered. For example, there is no first or last element.
- The elements of the set must be immutable objects. For example, a list cannot be an element of the set.
How to create a set
To create a set in Python, you can use the set() function and enclose the elements in square brackets.
For example, create a set of five numbers and assign it to the variable A.
>>> A = set([1,2,3,4,5])
Alternatively, you can create a set using a more concise syntax. The end result is the same.
>>> A = {1,2,3,4,5}
If you want to create an empty set, do not indicate any elements.
For example, type B = set() or B = {} to create an empty set in the variable B.
>>> B = set()
You can also insert elements of different types in a set, such as letters and numbers.
>>> A = {1,2,3,"a","b", "c"}
However, you cannot insert the same element multiple times in a set. If you do so, Python will still consider it only once.
For example, type A={1,2,3,3}
>>> A={1,2,3,3}
Then, display the contents of the set by typing A in the Python terminal.
In the set, the element 3 is present only once.
>>> A
{1, 2, 3}
Additionally, you cannot insert other sets inside a set of type set.
You can only insert single elements or, possibly, frozenset objects, a kind of unmodifiable set.
How to add elements to a set
To add a new element to a set, use the add() method.
For example, create a set of five numbers in the variable A.
>>> A = {1,2,3,4,5}
Type the command A.add(6) to insert the number 6 into the set.
>>> A.add(6)
Display the contents of the variable A.
Now the set A is composed of six elements.
>>> A
{1, 2, 3, 4, 5, 6}
How to remove elements from a set
To remove an element from a set, you can use three methods: discard(), remove(), and pop().
For example, create a set of five letters in the variable A.
>>> A = {"a","b","c","d","e"}
Now try to use one of these methods:
1] The discard() method
The discard() method removes an element without returning anything.
Type the command A.discard("e") to remove the element "e" from the set A.
>>> A.discard("e")
Display the contents of the variable A.
Now the set A is composed of four elements.
>>> A
{'a', 'b', 'c', 'd'}
If the discard() method does not find the element in the set, it does not raise any exceptions.
2] The remove() method
The remove() method removes an element from the set without returning anything, just like the discard() method. However, it raises an error if the element to remove is not found.
To remove the element "c" from the set A = {"a", "b", "c", "d"}, simply enter the command A.remove("c")
>>> A.remove("c")
Then, display the contents of the variable A.
The set A now has three elements.
>>> A
{'a', 'b', 'd'}
If the remove() method cannot find the element in the set, it raises an exception.
3] The pop() Method
The pop() method retrieves a random element from the set and removes it.
To remove an element from the set A = {"a", "b", "d"}, simply enter the command A.pop()
>>> element = A.pop()
Then, display the contents of the variable A.
The set A now has two elements.
>>> A
{'a', 'd'}
The retrieved element is stored in the variable element.
To display the value of element, type element in the Python terminal and press enter.
>>> element
'b'
The element 'b' is stored in the variable element.
Note that in this case, you cannot choose which element to remove from the set. Python randomly selects the element to return.
The pop() method only raises an exception if you try to retrieve an element from an empty set.