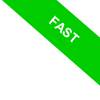
Enumerations in Python
Enumerations in Python provide a predefined set of values that help make your code more readable and reduce the risk of errors.
Imagine having a limited set of choices, like the days of the week or traffic light colors. Instead of using plain strings or numbers to represent them—which could easily lead to mistakes—you can use an enumeration.
Python offers a dedicated class called `Enum` that allows you to define enumerations. This class is available in the `Enum` module.
Let’s consider a practical example. Say you want to represent the days of the week.
Without using the `Enum` class, you might write something like this:
day1 = "Monday"
day2 = "Tuesday"
day3 = "Wednesday"
That looks fine, right? But what if someone writes "Wednesdáy" with an accent, or "Thursdy" with a typo?
This introduces the possibility of errors—exactly the kind of problems that enumerations can help you avoid.
Now, let’s see how using the Enum class can improve this.
With `Enum`, you can define the days of the week in a much safer and structured way:
from enum import Enum
class DaysOfWeek(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 4
FRIDAY = 5
SATURDAY = 6
SUNDAY = 7
In this example, you’ve created a `DaysOfWeek` class that inherits from `Enum`.
Each day has a clearly defined name and an associated numeric value, allowing you to reference days of the week without worrying about typos:
today = DaysOfWeek.MONDAY
print(today)
This will print:
DaysOfWeek.MONDAY
You can also access the numeric value associated with each day:
print(today.value)
1
Now, let’s take another practical example. Imagine you’re building a program to control traffic lights.
You could use strings like `"red"`, `"yellow"`, and `"green"`, but what happens if someone accidentally types `"greeen"`?
With an Enum, you can clearly define the valid values:
from enum import Enum
class TrafficLight(Enum):
RED = 1
YELLOW = 2
GREEN = 3
def traffic_light_action(color):
if color == TrafficLight.RED:
print("Stop!")
elif color == TrafficLight.YELLOW:
print("Prepare to stop.")
elif color == TrafficLight.GREEN:
print("Go!")
else:
print("Invalid color.")
traffic_light_action(TrafficLight.RED)
In this example, the function `traffic_light_action` is designed to receive a value (called `color`) from one of the members of the TrafficLight enumeration.
Automatic Naming
Here’s a useful trick when working with enumerations.
If you don’t need to assign specific values to the members of an Enum, you can use `auto()` to let Python handle it for you:
from enum import Enum, auto
class TrafficLight(Enum):
RED = auto()
YELLOW = auto()
GREEN = auto()
In this case, values are automatically assigned (0, 1, 2, and so on), without requiring manual input.
Why Are Enumerations Useful?
First, using `Enum` ensures that only the correct values are passed into your functions, reducing the risk of errors.
Another benefit is that you can easily iterate over an Enum to get all its members.
For example, if you want to print all the traffic light colors:
for color in TrafficLight:
print(color)
This will print:
TrafficLight.RED
TrafficLight.YELLOW
TrafficLight.GREEN
Another important aspect: when using enumerations, you don’t compare values the same way you would with strings or numbers.
Instead of comparing `TrafficLight.RED` directly with `1`, you compare enumeration members to each other. This provides an extra layer of safety to avoid accidental mistakes.
color = TrafficLight.RED
if color == TrafficLight.RED:
print("The color is red!")
In conclusion, enumerations are an incredibly useful tool for managing a fixed set of values. They make your code more readable, more reliable, and less error-prone.
While you could manage everything with strings and numbers, why risk it when Python offers a structured approach with `Enum`?
So, next time you need to represent a defined set of states, consider using enumerations.