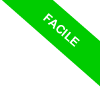
Python Dictionaries
When it comes to Python programming, dictionaries are an indispensable part of the language. They are powerful data structures that consist of key-value pairs, allowing for efficient mapping.
Crafting a dictionary in Python requires you to encapsulate these pairs within curly braces, neatly separated by commas, like so:
dictionary = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
Here, 'key1', 'key2', and 'key3' serve as the unique identifiers, the keys, while 'value1', 'value2', and 'value3' are the corresponding values.
This uniqueness of keys is a fundamental aspect of dictionaries. Each key acts as a special passcode, unlocking access to its associated value.
You can also create a dictionary using the dict() class by passing an iterable object that contains pairs of elements. For example, using a list of tuples:
dict([('key1', 'value1'), ('key2', 'value2')])
Alternatively, you can use key-value notation:
dict(key1='value1', key2='value2')
In both cases, the dictionary is formed by mapping the specified keys to their corresponding values.
In Python dictionaries, the search operation is performed based on keys. This is because dictionaries, unlike their fellow data structures like lists and tuples, do not maintain an inherent order of their elements. The beauty of this design can be understood by comparing it with a telephone directory. In it, you'd look up a friend's name (the key), instead of memorizing their number (the value). A Python dictionary operates in a remarkably similar fashion.
Let's delve into an example for a clearer understanding.
Consider this dictionary, named "countries":
countries = {
'UK': 'United Kingdom',
'FR': 'France',
'DE': 'Germany',
}
To retrieve a value from this dictionary, simply use the corresponding key.
For example, to retrieve the value for "DE", you would enter: countries['DE']
print(countries['DE'])
When you run: print(countries['DE']), Python scours the "countries" dictionary for the 'DE' key and fetches the corresponding value – "Germany".
Germany
But what if we search for a key that doesn't exist in our dictionary?
In such cases, Python responds with a KeyError exception.
Suppose you try to access the key "IT".
print(countries['IT'])
Python wouldn't find this key in the dictionary and would thus return an error message: KeyError: 'IT'
KeyError: 'IT'
Fortunately, Python provides a method called get() to handle such situations more gracefully. It returns "None" or a default value when the key doesn't exist.
print(countries.get('IT'))
For instance, running print(countries.get('IT')) still won't find the key but it will return "None", instead of throwing an error.
None
You can also customize the default return value for unsuccessful searches.
This can be achieved by adding a second parameter to the get() method:
print(countries.get('IT', 'does not exist'))
In this scenario, the unsuccessful search for the "IT" key will return the string "does not exist".
does not exist
So, how do we modify or add items in the dictionary?
Being mutable objects, dictionaries allow items to be added, removed, or changed after they have been created.
To do this, simply use the assignment operator =
.
Let's illustrate this with a practical example.
countries['IT'] = 'Italia'
With this line of code, we've added the "IT" key and its associated value "Italia" to the dictionary.
Now our dictionary comprises four items..
print(countries)
{'UK': 'United Kingdom', 'FR': 'France', 'DE': 'Germany', 'IT': 'Italia'}
Moreover, the same syntax can be used to modify the value associated with an existing key.
For example, to alter the value of the "IT" key from "Italia" to "Italy", we would use:
countries['IT'] = 'Italy'
And just like that, the value of the existing key is modified, without adding another item to the dictionary.
print(countries)
{'UK': 'United Kingdom', 'FR': 'France', 'DE': 'Germany', 'IT': 'Italy'}
But what about removing an item from the dictionary?
For this, Python offers the del statement.
del countries['IT']
The command: del countries['IT'] removes the "IT" key and its associated value from the "countries" dictionary.
print(countries)
{'UK': 'United Kingdom', 'FR': 'France', 'DE': 'Germany'}
And there you have it! Now you have the ability to delete any existing key from your dictionary.
Python equips you with a multitude of methods to efficiently work with dictionaries. The keys() method returns all the keys, the values() method fetches all the values, and the items() method displays all key-value pairs as tuples. Each of these methods will be explored in-depth in forthcoming tutorials.