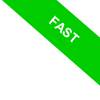
The __delitem__() Method in Python
In Python, the special method `__delitem__()` is used to define what happens when you remove an item from an indexed data structure, such as a list or a dictionary.
object.__delitem__(index)
Simply put, when you use the `del` statement to delete an item from a list or a key from a dictionary, Python automatically calls the `__delitem__()` method.
For example, let's create a list with five elements:
numbers = [10, 20, 30, 40, 50]
Now, let's delete the third element from the 'numbers' list using the del statement.
Remember, list indices start at zero, so the third element has an index of 2.
del numbers[2]
When you run `del numbers[2]`, Python internally calls the `__delitem__()` method on the 'numbers' object with index 2, which is equivalent to writing 'numbers.__delitem__(2)'.
This means you could achieve the same result by writing numbers.__delitem__(2)
numbers.__delitem__(2)
Now, the list has one less element:
print(numbers)
[10, 20, 40, 50]
As we'll explore in the next section, the `__delitem__()` method is also quite useful when you're creating custom data structures.
Customizing a Class with the `__delitem__()` Method
The `__delitem__()` method also allows you to create custom data structures and modify how objects behave when they need to act like indexed containers (e.g., lists, dictionaries, tuples, etc.).
Here's a practical example.
Imagine you have a class called `MyDictionary` that you want to behave similarly to a Python dictionary but with some additional functionality.
You can define the `__delitem__()` method to control how items are deleted.
class MyDictionary:
def __init__(self):
self.data = {}
def __setitem__(self, key, value):
self.data[key] = value
def __getitem__(self, key):
return self.data[key]
def __delitem__(self, key):
print(f"Deleting key: {key}")
del self.data[key]
Create a `MyDictionary` object, which initializes an internal empty dictionary (`self.data`).
my_dict = MyDictionary()
When you add an item with `my_dict['a'] = 1`, Python calls the `__setitem__()` method we defined, and the key-value pair is added to the internal dictionary.
my_dict['a'] = 1
Add another item as well:
my_dict['b'] = 2
If you now access an item with `my_dict['a']`, Python calls the `__getitem__()` method and returns the value associated with that key.
print(my_dict['a'])
1
When you use `del my_dict['a']`, Python calls the class's `__delitem__()` method.
del my_dict['a'] #
Deleting key: a
In this case, the method not only deletes the item but also prints a message to let you know what's happening.
Why use `__delitem__()`? This method is useful when you want greater control over what happens when items are removed from a data structure. For instance, you might update other data structures, perform cleanup tasks, or even prevent the removal of items if certain conditions aren't met.
I hope this explanation was helpful! If you have any further questions or want to explore another topic, feel free to leave a comment below.