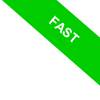
Python's Garbage Collection
Python, a powerful programming language, employs an automated memory management mechanism known as garbage collection. Its primary role? To cut the ties to objects that have outlived their usefulness in the program, effectively freeing up the memory they once hogged.
This unassuming yet crucial process allows for a more efficient use of resources and forms a protective barrier against memory overflow, an issue that rears its head when a program continues to hoard memory without setting it free.
Example
Let's explore this with an example to help drive the point home.
Take the integer value 77, for instance. Let's assign it to a variable 'x'.
x=77
What we've done here is forged a link between our variable's name 'x' (the label), and the integer value 77 (the object).
However, bear in mind that the label 'x' doesn't house the value 77 per se. Rather, it holds a reference to the memory address where the value 77 resides.
Now, let's shake things up a bit and assign a new value to 'x'.
x=10
This action severs the previous link between 'x' and 77 and establishes a fresh bond between 'x' and the new object, 10.
This new object resides at a different memory address from its predecessor.
Our initial object, 77, is now without a label referencing it, effectively rendering it unused in our program.
This is where our unsung hero, the garbage collector, springs into action, wiping the object "77" from memory and liberating the space it once consumed.
This nifty process allows for an increase in the amount of memory available for data storage and safeguards against the peril of obsolete data overwhelming the available memory space.
A Deep Dive into Python's Garbage Collection Mechanism
Python's memory management strategy is two-pronged, harnessing the power of reference counting and cyclic garbage collection.
1] Reference Counting
This strategy forms the backbone of Python's memory management mechanism.
- +1 reference
Each time an object receives a reference - when it's assigned to a variable or added to a list, Python gives the reference count of that object a one-up. - -1 reference
And when an object's reference is removed, Python dials down the reference count by one.
When an object's reference count dwindles to zero, it's a clear sign that the object has no ties left within the program.
And that's the garbage collector's cue to safely liberate the memory that was previously claimed by that object.
2] Cyclic Garbage Collector
Python periodically unleashes a cyclic garbage collection.
This is a dedicated component of Python's memory management system that seeks out and handles reference cycles, liberating memory that would otherwise lay fallow.
A reference cycle crops up when two or more objects hold mutual references, yet lie dormant and unused by the rest of the program.
Consider, for example, this code that conjures up two empty lists, each holding a reference to the other - a classic "cycle" of references.
list1 = []
list2 = []
list1.append(list2)
list2.append(list1)
Although this scenario evades detection via reference counting (since none of the objects' reference counts have plummeted to zero), these two objects aren't truly active in the program.
The cyclic garbage collector was designed to detect such elusive scenarios and snuff them out.
In conclusion, Python's masterful combination of reference counting and the cyclic garbage collector makes for an efficient, safe, and effective memory management mechanism. It ensures that memory space is released when its service is no longer needed and remains on standby for new objects when the need arises.