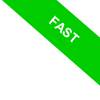
Python arrays
Arrays are one of the most widely known and used tools across all programming languages.
What is an array? Think of it as a series of organized boxes where you can store whatever you like. You can access, modify, and even perform operations on them. Essentially, an array is a data structure that holds an ordered collection of elements, all of the same type (such as integers, floats, etc.), accessible via a numerical index.
In Python, there isn't a built-in array data structure like in some other languages. Instead, there’s something similar—and perhaps more versatile—called lists.
However, if you need to define a true array, you can use the Array module or NumPy.
Lists in Python
Lists are similar to arrays, but with one key difference: they can hold values of different types.
This means a list could have an integer in one box, a string in another, and even another list in a third box.
For example, here’s a list of integers:
numbers = [1, 2, 3, 4, 5]
But you can also create a list with mixed types:
mixed = [2020, "hello", 3.14, [1, 2, 3]]
You can access individual elements by specifying their position within square brackets.
For instance, to access the first element of the 'mixed' list, you would write mixed[0]:
print(mixed[0])
2020
Remember, in Python, indexing starts at zero.
So, to access the second element, you’d write mixed[1], and so on.
print(mixed[1])
hello
Unlike traditional arrays, lists offer much more flexibility. They’re far more versatile.
However, if you're working with large amounts of numerical data, I recommend using more specialized tools like arrays from the 'array' or 'NumPy' libraries.
Arrays with the `array` module
If you're looking for something closer to traditional arrays, Python offers the array module, which allows you to create arrays where all elements must be of the same type (similar to lower-level languages like C or Java).
The advantage? These data structures are more memory-efficient and provide better performance when handling large datasets.
Here’s an example of an integer array using the `array` module:
First, you’ll need to import the array module:
import array
Then, you can create any array you need.
For example, let's create an array of integers and store it in the variable 'numbers':
numbers = array.array('i', [1, 2, 3, 4, 5])
Here, the `'i'` specifies that the array will only contain integers (`int`).
Each data type has its own specific code in the array module (for example, `'f'` for floats).
print(numbers)
array('i', [1, 2, 3, 4, 5])
Just like with lists, you can access the elements of an array using their indices.
Indices are simply numbers that represent the position of each element in the array, starting from 0 (so the first box is number 0).
For example, to access the third element of the array, you’d write:
print(numbers[2])
3
You can also modify the values in an array just as you would with a list:
numbers[1] = 10
Now, the array has the value 10 in the second position.
print(numbers)
array('i', [1, 10, 3, 4, 5])
When should you use arrays?
You might wonder, "When should I use an array instead of a list?"
If you’re working with large volumes of numerical data and need efficient memory usage, arrays are the ideal choice.
For instance, when handling hundreds of thousands of numbers, arrays from the `array` module—or better yet, NumPy arrays (which are more powerful)—are far more efficient than lists.
NumPy Arrays
If you need to perform serious mathematical operations on arrays, I highly recommend NumPy, an external library that provides much more efficient and powerful multidimensional arrays than Python’s built-in lists or arrays.
Here’s how to create an array with NumPy:
First, import the NumPy library:
import numpy as np
Then, create an array using NumPy’s array method:
numbers = np.array([1, 2, 3, 4, 5])
This gives you a vector of numerical values.
print(numbers)
[1 2 3 4 5]
Unlike lists and basic arrays, NumPy allows you to perform vectorized operations quickly and efficiently.
For example, you can multiply every element of the array by two in a single step!
numbers = numbers * 2
print(numbers)
[ 2 4 6 8 10]
This is much more efficient and convenient when working with large datasets.
Additionally, you can also create multidimensional arrays.
For example, let’s create a 3x3 matrix:
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
This gives you a two-dimensional array with three rows and three columns:
print(matrix)
[[1 2 3]
[4 5 6]
[7 8 9]]
You can access elements within this matrix using indices, just as you would with a list of lists.
For example, to access the second element of the first row, you would write:
print(matrix[0, 1])
2
This is just a simple example of what NumPy can do.
If you work with matrices or linear algebra frequently, NumPy is an indispensable tool in Python.
It makes handling matrices and performing vector or matrix operations much more