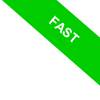
The __setitem__() Method in Python
The '__setitem__()' method in Python is a special method that's triggered when you use square bracket syntax to assign a value to an element within a data structure, such as a dictionary or a list.
object.__setitem__()
Let's look at a practical example.
When you write something like this in a dictionary:
my_dict['key'] = 'value'
What happens behind the scenes is that Python automatically invokes the '__setitem__()' method on the 'my_dict' object, passing 'key' as the first argument (the key) and 'value' as the second argument (the value to be associated with the key).
In general, the '__setitem__()' method is implemented in classes like 'dict' (dictionaries), 'list' (lists), and in any data structures that support accessing and modifying elements via a key or index.
You can also implement this method in your own classes to allow your objects to behave similarly to built-in data types.
For instance, if you create a class that needs to function like a dictionary, you can define the '__setitem__()' method to manage how values are assigned to keys.
A Practical Example
Imagine you want to create a class called 'AddressBook' that stores phone numbers associated with people's names, similar to how a dictionary works.
In this data structure, you should be able to add phone numbers and names using square bracket syntax.
Here’s how you can achieve that:
class AddressBook:
def __init__(self):
self.contacts = {}
def __setitem__(self, name, number):
self.contacts[name] = number
print(f'Added {name} with number {number}')
def __getitem__(self, name):
return self.contacts.get(name, 'Contact not found')
In the AddressBook class, we’ve defined two methods:
- The __setitem__() method takes the person's name and phone number as arguments.
- The __getitem__() method retrieves phone numbers using square bracket syntax.
Next, let's create an instance of this class.
address_book = AddressBook()
Then, assign some values to the object:
address_book['Alice'] = '123-4567'
Added Alice with number 123-4567
When you write `address_book['Alice'] = '123-4567'`, Python calls the `__setitem__()` method of the `AddressBook` class, passing 'Alice' and '123-4567' as arguments.
This means you could achieve the same result by writing:
address_book.__setitem__('Alice', '123-4567')
In both cases, the key and value are stored.
Now, let’s add another key-value pair to the 'address_book' object:
address_book['Bob'] = '987-6543'
Added Bob with number 987-6543
At this point, the structure contains two key-value pairs.
You can retrieve the information by specifying the key within square brackets:
print(address_book['Alice'])
This command indirectly calls the address_book.__getitem__('Alice') method and displays the value associated with the 'Alice' key.
123-4567
In summary, defining the '__setitem__()' method allows you to customize how your class handles value assignments.
This can be especially useful if you want to perform additional operations whenever a value is added or modified, such as validating data, logging the action, or even updating a user interface.
I hope this explanation has clarified how this method works and why it's beneficial!