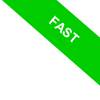
Local Namespaces in Python
In Python, a "local namespace" refers to a space that encompasses names like variables, functions, and classes, which are defined within a function, an object, or a certain segment of code.
What makes it local? This designation as "local" stems from its relevance and existence solely within the confines of the function where it's defined. Beyond this scope, such names lack recognition and accessibility. This principle extends to methods, classes, and list comprehensions, making the local namespace a pivotal concept among Python's namespaces.
Executing a function prompts Python to establish a local namespace for it, ensuring that any variables assigned within the function are confined to this segregated space.
If you attempt to access a variable defined in a function from another function or the global scope, Python will indicate that the name is unknown.
Once a function concludes, Python disposes of the local namespace.
This mechanism effectively isolates variables defined in a function, safeguarding them from external interference and positioning functions as self-contained units that veil their internals from the external environment.
To concretely grasp how local namespaces function in Python, let's explore a hands-on example.
Consider writing a program that calculates the square of a number, then leverages this outcome for an additional computation.
def calculate_square(number):
result = number ** 2
return result
number = 4
y=calculate_square(number)
print(y)
Here, the calculate_square() function accepts a number, calculates its square, and returns the result. The `result` variable, established within the calculate_square() function, resides solely in that function's local namespace as a local variable.
Following the function's execution, its local namespace is eliminated, erasing all within it from the program's remainder.
16
Conversely, the `number` variable falls within the global namespace as it is set at the script's highest level, showcasing Python's handling of local namespaces.
Recursive Functions
In the realm of recursive functions, Python establishes a local namespace for each invocation. This approach ensures that each function accesses its own set of local variables, thereby avoiding any conflict with variables in other namespaces.
Defining Recursive Functions Simply put, a recursive function is one that calls itself as part of its execution process. This method of solving problems by breaking them down into smaller, manageable subproblems of a similar nature is often highly efficient.
Take the factorial calculation as a prime example of recursion in action. The factorial of a number (n!), represents this concept perfectly.
def factorial(n):
# Base case: when n is 0, factorial equals 1
if n == 0:
return 1
# Recursive step: calculating n! as n * (n-1)!
else:
return n * factorial(n-1)
# Demonstrating function usage
num = 5
print(f"The factorial of {num} is: {factorial(num)}")
Through a sequence of nested calls, each with a decrementing 'n' value until it reaches 0, recursion unfolds. Subsequently, the results are compiled back to the initial call, layer by layer.
For every call made, Python crafts a unique local namespace, assigning a distinct 'n' value in each.
As functions complete their execution, Python dismantles the local namespace, ultimately delivering the final outcome.
The factorial of 5 is: 120
This outcome is 120 because the factorial of 5! is the multiplication of 5·4·3·2·1, equating to 120.
Utilizing the locals() Function
The locals() function returns a dictionary that maps the symbol table of the current local namespace.
print(locals())
Calling locals() within a function reveals all variables defined within that specific function. This feature proves invaluable for debugging and introspection, offering a snapshot of accessible variables and their values at any given point.
Invoking the locals() function at a module's top level, outside any function, yields the dictionary of the module's global namespace.
Another tool for accessing the local namespace is the vars() function.
print(vars())
Like locals(), vars() also presents the local namespace as a dictionary.