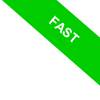
The globals() Function in Python
The globals() function is a gateway to Python's global namespace, offering direct access to its labels.
globals()
Invoking the globals() function in Python hands you back a dictionary that serves as a map, linking each global name to its corresponding value.
This function generates a dictionary encompassing all names declared globally within your program, covering everything that can hold a value: from variables and functions to classes and modules.
Within this dictionary lies a comprehensive overview of the program's global namespace, enabling you to not only view but also amend every global variable and its value. This opens up possibilities not just for inspection but for dynamic adjustments to the global names and their values.
Consider defining a couple of variables as a starting point.
x = 5
y = "Hello, world!"
Let's peek into the global dictionary.
print(globals())
This action reveals the contents of the global dictionary, showcasing `x`, `y`, and everything else established at the global level.
{'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x7f979d377c10>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': '/home/main.py', '__cached__': None, 'x': 5, 'y': 'Hello, world!'}
Beyond the variables you've personally defined, the dictionary also includes numerous names established by Python itself or through imported modules.
Altering Variables with globals()
Moving onto a more compelling application: altering a global variable through globals().
globals()['x'] = 10
And just like that, `x` shifts to 10.
print(x)
10
By employing `globals()['x'] = 10`, the global variable `x` transitions from `5` to `10`, bypassing the need for direct reference to `x`.
This illustrates the efficacy of the global dictionary in facilitating modifications.
Embarking on another example, let's imagine a scenario where your program initiates with a set of global variables but necessitates the inclusion of new ones dynamically as it runs.
Initiate by defining a few global variables
variable1 = "I am a global variable"
variable2 = 42
Next, dynamically introduce a new global variable
globals()['variable3'] = "This was added dynamically!"
In this scenario, `variable1` and `variable2` are traditionally initialized, while `variable3` is dynamically woven into the global namespace using globals().
This showcases the versatility and power of the globals() function in dynamically manipulating your program's global namespace.
Yet, a word of caution: while globals() is a potent tool for state modification, it demands prudent use. Its power comes with risks, potentially complicating code readability and comprehension. It's advised to reserve such a powerful feature for occasions where its necessity is unequivocal, like in metaprogramming or when dealing with complex dynamic situations.