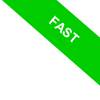
Python's Built-in Namespace
The built-in namespace in Python holds a special place because it includes built-in functions and types readily available to developers, without the need for imports. This namespace is accessible throughout the Python interpreter's lifecycle.
Beyond essential functions like `print()`, `id`, and `len()`, it also encompasses fundamental data types such as `int`, `float`, `dict`, and `list`.
Understanding Built-ins
Python offers a suite of pre-configured functions and types that you can use in any project right out of the box. These built-ins are essential for a variety of common tasks, for example:
- Functions: `print()`, `len()`, `type()`, `id()`, `range()`, and more.
- Types: `int`, `float`, `dict`, `list`, `tuple`, `set`, `str`, among others.
- Exceptions: `Exception`, `ValueError`, `TypeError`, `KeyError`, and so on.
These tools are always within reach because they're part of the built-in namespace.
Try executing these commands in the Python terminal for a hands-on example:
numbers = [1, 2, 3, 4]
# Using the built-in 'len' function
print(len(numbers))
There's no need to import the `print` function; it's predefined and loads automatically with the Python session.
4
This principle applies to types as well. For instance, the integer type 'int' is a basic data type provided by the language.
number = 10
To dive deeper into the built-in namespace, explore the `builtins` module:
import builtins
# Display all built-in functions and types
print(dir(builtins))
This list shows everything at your disposal without any additional imports.
Best Practices: Avoid Overwriting Built-ins
Python's flexibility allows you to tailor your programming experience. However, overwriting a built-in function or type can lead to confusion and errors:
Consider this example where the `print` function is overwritten:
def print(message):
return f"Overwritten message: {message}"
While Python permits this, you'll temporarily lose access to the original `print` function, affecting your session or script's functionality.
To revert to the standard behavior, you'll need to delete the custom definition or restart the Python interpreter.
Therefore, while customization is possible, changing or replacing built-ins should be done cautiously and only when absolutely necessary.