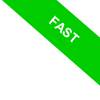
Global Keyword in Python
The global keyword in Python is a powerful feature that allows you to reference variables defined outside of the current function or scope, making them accessible throughout your entire program.
global variableName
This feature proves invaluable when you're looking to maintain and update a value that plays a critical role in various sections of your code.
Let's delve into a concrete example to illustrate this concept.
Imagine we're keeping track of how many times a specific resource is accessed within our program. We intend to increment a counter each time this resource is utilized.
access_counter = 0
def resource_access():
global access_counter
access_counter += 1
print(f"Resource accessed: {access_counter}")
resource_access()
resource_access()
By declaring `global access_counter`, we instruct Python to treat `access_counter` as a global variable, not a local one.
This approach allows us to modify `access_counter` defined outside our function without the need to initialize a new local variable under the same name.
Thus, we can seamlessly update the access count throughout the lifespan of our program.
The program yields the following output:
Resource accessed: 1
Resource accessed: 2
Although the `global` keyword is incredibly useful, it's wise to exercise restraint in its application.
Excessive use of global variables can obscure your code, given that any part of the code can alter these variables. This can make it challenging to track down and debug the source of errors.
It's recommended to resort to global variables only when it's strictly necessary.
Considering Alternatives to Global Variables. Opt for classes or passing variables between functions to minimize your dependency on global variables. Should the use of global variables be unavoidable, ensure their role and application within the code are thoroughly documented. This practice aids in enhancing the code's clarity and maintainability, benefiting not just you but also your fellow developers in navigating the program's logic.
This overview aims to enrich your comprehension of when and how to effectively employ the `global` keyword within your Python projects.