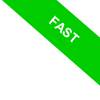
Scope in Python
Let's explore the concept of "scope" in Python, an essential element in programming that determines the visibility of a variable within different parts of your code.
The term "scope" refers to the contextual boundary within which a variable is both defined and accessible. It plays a critical role in the structure and organization of code, influencing both readability and maintainability.
Python employs a straightforward approach to scope resolution through the LEGB rule, which stands for Local, Enclosing, Global, and Built-in, each representing a different level of variable accessibility:
- Local (L)
The most specific level where variables are defined within a function and are only accessible within that function's body, ensuring a tight-knit and secure environment for variables. - Enclosing (E)
Pertains to the environment of outer functions, where variables remain accessible to their inner functions, facilitating a hierarchical structure that promotes clarity and efficiency in nested functions. - Global (G)
Encompasses variables defined at the module's top level or declared global within a function, offering a broader reach across your code base. - Built-in (B)
The broadest scope, encompassing Python's built-in names, allowing these predefined names to be globally accessible and laying the foundation for the language's functionality.
To illustrate these concepts further, let's look at practical examples.
Example of Local Scope
Consider the variable "local_variable"; it is only accessible within the confines of its function, illustrating the principle of local scope.
def my_function():
local_variable = "I'm only visible inside this function."
print(local_variable)
my_function()
I'm only visible inside this function.
Attempting to access "local_variable" outside its function would result in an error, highlighting the encapsulated nature of local scope.
Global Scope Example
Conversely, "global_variable" demonstrates global scope, being accessible throughout the entire program, including within functions.
global_variable = "I'm visible across the entire program."
def my_function():
print(global_variable)
my_function()
I'm visible across the entire program.
This global accessibility contrasts with local variables, which are confined to their respective functions.
Significantly, global variables can be modified from any part of the program, a feature illustrated in the following example.
global_variable = "Original"
def modify_global():
global global_variable
global_variable = "Modified"
print(global_variable)
modify_global()
print(global_variable)
An Example of Enclosing Scope
Enclosing scope refers to the scope that surrounds (or "encapsulates") a local scope within a nested function.
This is the scope found between the local and global scopes, accessible by inner functions but not from outside the container function.
In essence, it allows nested functions to access non-local variables of their "parent" function where they're defined.
For instance, this script features two nested functions.
def outer_function():
enclosing_variable = "Visible within the inner function."
def inner_function():
print(enclosing_variable)
inner_function()
outer_function()
The outer (parent) function calls the inner function, which can access all variables of the parent function.
In this case, the outer function prints the content of the "enclosing_variable".
Visible within the inner function.
An Example of Built-in Scope
Built-in scope in Python refers to a level of scope that contains names of functions, keywords, and pre-defined variables built into the Python language itself.
These are accessible from anywhere in your code without needing to import or define them explicitly.
Here's a simple example:
my_list = [1, 2, 3, 4, 5]
list_length = len(my_list)
print(list_length)
In this script, we use the built-in function len() to get the length of a list.
Then we use the built-in function print() to display a value.
5
In this example, both `len()` and `print()` are built-in functions of Python. You don't need to import them from any module to use them; they are always available anywhere in your code. This is a great example of how the built-in scope works in Python.
These examples illustrate how Python handles the "scope" of variables.
Understanding these rules is crucial for writing clear code and avoiding errors related to variable visibility.