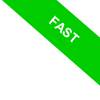
Local Variables in Python
In Python, local variables refer to variables that are defined within a function.
The scope of a local variable is limited to the function in which it is defined, meaning it cannot be accessed outside of that function.
Example:
Consider the variable x as a local variable within the function func()
x=2
def func():
x=5
func()
print(x)
The output of this program will be 2. This is because the assignment of the local variable x to 5 within func() does not impact the value of x in the rest of the program.
2
The value assigned to the local variable (x=5) can only be accessed within the specific function func() where it was defined.
To use a function's local variable elsewhere in your program, you must promote it to a global variable using the global keyword.
x=2
def func():
global x
x=5
func()
print(x)
This time, the output will be 5, because the variable x within func() is now a global variable, allowing the assignment x=5 to overwrite the initial x=2.
5