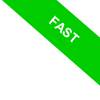
Python's nonlocal statement
The `nonlocal` keyword in Python is a bit of a niche tool, but it's incredibly handy once you get the hang of it. Essentially, it allows you to flag a variable in a nested function as not local, meaning Python will treat it as belonging to the nearest enclosing scope outside the nested function.
nonlocal variablename
This comes into play when you're working within nested functions and you need to modify a variable that's not in the local scope of your current function but also not global.
It's a way to say, "Hey Python, this variable I'm working with belongs to an outer function, not this one, nor is it a global variable."
Without the `nonlocal` declaration, if you try to assign a value to a variable that's recognized in an outer scope, Python would typically create a new local variable within your nested function, which isn't always what you want. `nonlocal` gives you the control to directly modify the variable in its original scope.
Let's dive into an example to clear things up.
In the following script, we have a pair of nested functions:
def outer():
var = "outer value"
def inner():
nonlocal var
var = "modified value"
inner()
print(var)
outer()
By leveraging `nonlocal` within the inner() function, we can successfully update `var` from "outer value" to "modified value" — a change that reflects outside the inner function, all the way up to the outer function's scope.
It's a subtle but powerful way to manage variable scope in more complex functions.
Therefore, the final result is as follows
modified value
Remember, `nonlocal` is specifically designed for use within nested functions.
It's not applicable for global variables or for functions that aren't enclosed within another function.
Also, the variable you're declaring as `nonlocal` must already exist in the enclosing function's scope—it can't be introduced as `nonlocal` without a prior definition.
If Python can't find a matching variable in any enclosing scopes, it'll throw an error, signaling that it couldn't find an appropriate variable to apply `nonlocal` to.
Take this script as a case in point: here, `var` is initially defined in the global scope. Attempting to use `nonlocal` in such a scenario will result in an error because Python expects `nonlocal` variables to exist in an outer, but not global, scope.
def outer():
def inner():
nonlocal var
var = "modified value"
inner()
var = "outer value"
outer()
print(var)
SyntaxError: no binding for nonlocal 'var' found
For variables defined in the global scope, you're better off using the `global` keyword if you need to modify them from within a nested function.
def outer():
def inner():
global var
var = "modified value"
inner()
var = "outer value"
outer()
print(var)
modified value