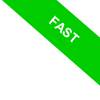
Python Namespaces
In programming with Python, you label things like variables or functions to reference them. These labels are stored in a "Namespace", a critical structure that helps Python organize and locate names efficiently in a program or session.
Simply put, namespaces ensure that each name used in your code is unique and can be identified without ambiguity, promoting clarity and order in your codebase.
Python namespaces come in three main flavors:
- Built-in
These names are preloaded into Python and available for use at any time. Functions like `print()` and `len()` fall into this category. - Global
Names defined at the top level of your code, outside of any specific functions or classes, belong here. They can be accessed from anywhere within your program. - Local
These are names defined within a function or block of code and are only accessible in their specific context. Also includes the Enclosed namespace.
Python employs a set of rules, known as "name resolution", to determine which namespace to use when a name is referenced. This ensures that names are matched with their correct values.
Bear in mind, Python follows a specific order when resolving names: it first checks the local scope, moves on to the global scope, and finally turns to the built-in scope. This hierarchy, known as the "LEGB Rule" (Local, Enclosed, Global, Built-in), is designed to prevent naming conflicts and maintain a well-structured codebase.
Given that a label can exist in multiple namespaces, each one is tied to a "scope" indicating its point of origin. This tells Python where to begin its search for the correct reference.
For instance, a variable defined inside a function has a local scope and is inaccessible outside that function. In contrast, a global variable is available throughout the entire program.
Let’s explore a simple example to better understand these concepts in action.
We start by defining a global variable named `number` and assign it a value of `5`. This variable is accessible throughout our program.
number = 5
Then, we introduce a function called `example_function`.
Within this function, we declare another variable named `number` but assign it a value of `10`. This variable is local to the function.
number = 5
def example_function():
number = 10
print("Number inside the function:", number)
print("Global number before the function call:", number)
example_function()
print("Global number after the function call:", number)
This program features two variables named "number".
- One is globally scoped, available throughout the program.
- The other is locally scoped within the function.
Meanwhile, the `print()` function is a built-in Python label, predefined and ready to use.
What is the output?
Printing the "number" variable outside the function references the global variable `number`, displaying `5`.
Global number before the function call: 5
Within the function, the local variable `number` is used, showing `10`.
Number inside the function: 10
Following the function call, the global variable `number` is referenced again, returning to `5`.
Global number after the function call: 5
This example illustrates how variable "scope" – the context where a name is valid – can shift based on where it is defined within your code.
In summary, Python resolves the name "number" by checking the current scope first. If it doesn't find a match, it broadens the search to outer scopes, moving through the local, global, and built-in namespaces as needed.
This primer should have clarified the concepts of namespaces and scope in Python, showcasing their importance in writing clear and error-free code.