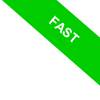
Python's locals() Function
The locals() function in Python serves as a window into the local variables within a function's scope. It's an invaluable tool for developers looking to debug or perform dynamic analysis of their code in real-time.
locals()
By integrating this function into your code, you can effortlessly inspect the current values of local variables at any point within a function. Its utility extends beyond mere debugging; it's a gateway to deeper code introspection during execution phases.
Specifically, locals() compiles a snapshot of the function's namespace, presenting it as a dictionary. Here, variable names serve as keys, with their respective values mapped accordingly.
A Practical Example
To demystify the workings of locals(), let's dive into a hands-on example.
Consider the following script:
def example():
a = 1
b = 2
c = 3
print(locals())
example()
This script crafts a function named "example()" which initializes three local variables and subsequently employs locals() to display the function's local namespace.
The resulting namespace is succinctly captured as:
{'a': 1, 'b': 2, 'c': 3}
This output reaffirms that locals() returns a dictionary mapping of local variables, enabling direct access or iteration over these key-value pairs.
While locals() finds its prime utility within function scopes, its application can extend to module-level contexts. However, for global namespace inspection, the use of globals() becomes more pertinent, offering a dictionary representation of the global symbol table.
One intriguing feature of `locals()` is its potential use for dynamically altering local variables. Yet, proceed with caution: tweaking the dictionary that locals() returns doesn't always adjust local variables as you might envisage. Thus, it's typically advised against.
def test_locals():
x = 10
print("Before:", locals())
locals()['x'] = 20
print("After:", locals())
print("The true value of x:", x)
test_locals()
You'll find that despite the apparent changes in the locals dictionary as shown by `locals()`, the "actual" value of `x` may remain unaffected.
This serves to highlight the limitations of attempting to manipulate local variables through locals().
Optimal Uses for locals()
The `locals()` function shines in debugging or logging scenarios, offering a quick snapshot of local variables at specific moments in your code. It's less ideal for modifying or dynamically interacting with these variables, as more straightforward and secure approaches usually exist.
In essence, locals() provides a powerful glimpse into the local variable landscape of Python. However, like any potent tool, it demands careful use and a deep understanding of its boundaries.
locals() in Object Methods
Extending its versatility, locals() can also be implemented within Python object methods. It's important to note, however, that within a method, locals() isolates the method's local variables rather than encompassing the entire object's scope.
This means that object instance variables fall outside the purview of locals() when used in a method context, thus not appearing in the returned dictionary.
For illustration, let's define a class:
class MyClass:
def __init__(self):
self.instance_variable = "This is an instance attribute"
def another_method(self):
another_local_variable = "This is another local variable"
def my_method(self):
local_variable = "This is a local variable"
print(locals())
Upon creating an instance and invoking the method that includes locals(), you'll observe:
object = MyClass()
object.my_method()
The output from locals() within "my_method()" exclusively lists the "local_variable".
{'self': <__main__.MyClass object at 0x7f00470ec2e0>, 'local_variable': 'This is a local variable'}
This demonstrates two critical aspects:
- 'self': Acts as a dictionary key, signifying the instance object on which the method is called, with the memory address indicating its allocation in memory.
- 'local_variable': A dictionary key denoting a method-specific local variable, illustrating the direct mapping of variable names to their values within the method.
It's evident that locals() strategically omits "another_local_variable" and "instance_variable" as they reside outside the method's local scope.
Accessing Instance Attributes
For accessing instance attributes or variables within a method, direct reference through the "self" prefix is the norm. For instance, "self.instance_variable" provides straightforward access.
Example:
class MyClass:
def __init__(self):
self.instance_variable = "This is an instance attribute"
def show_attribute(self):
# Accessing the instance attribute
print(self.instance_variable)
object = MyClass()
object.show_attribute()
This is an instance attribute
In sum, locals() is instrumental for local variable inspection within methods, whereas "self" is the conduit for object attribute access.