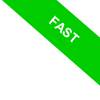
Python's vars() Function
The vars() function in Python is a gateway to an object's attributes or the variables currently in play within a namespace.
vars()
At its core, vars() reveals an object's `__dict__` attribute, essentially a dictionary brimming with the object's attributes and their values.
Using vars() without any arguments transforms it into a mirror of locals(), offering up a dictionary that maps out the local variables of the namespace you're working in.
Why it's a game-changer: vars() shines when it comes to the dynamic exploration or adjustment of an object's properties. Imagine needing to sift through an object's attributes, reading or tweaking their values. This is where vars() becomes invaluable, paving the way for more dynamic programming practices.
Let's dive into a concrete example:
Consider a class named `Car`, endowed with attributes like `make`, `model`, and `year`.
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
Next, instantiate the Car class:
my_car = Car("Fiat", "500", 2021)
To peek into `my_car`'s attributes, vars() is your tool of choice.
print(vars(my_car))
This command unfurls a dictionary akin to:
{'make': 'Fiat', 'model': '500', 'year': 2021}
Seamlessly Tweaking Attributes
Vars() isn't just for snooping around; it's also your ally in dynamically changing an object's attributes.
Take `my_car`, for instance:
car_attributes = vars(my_car)
Want to update the year? It's as simple as:
car_attributes['year'] = 2024
Query the object again, and Python proudly presents the updated value:
print(my_car.year)
2024
In summary, vars() is a robust tool for object inspection and manipulation within Python.
It offers a peek under the hood of objects, equipping you with the means to craft more dynamic and adaptable code. Yet, it's crucial to remember that vars() is only applicable to objects with a `__dict__` attribute, which covers a broad spectrum of user-defined objects.
Not every Python object comes with a `__dict__` attribute, though.
For instance, attempting vars(42) in the Python console will lead to an error, as integers lack the attributes for such examination or modification.