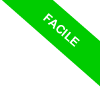
Python's bin() Function
Python boasts a diverse set of built-in functions, and among them is the bin() function. Its primary role is to transform an integer into its binary string equivalent.
bin(x)
In this context, `x` represents an integer.
The function yields a string that mirrors the number's binary representation in base 2.
Notably, in Python, binary strings are prefixed with "0b", denoting their binary nature. These strings are composed solely of the digits 0 and 1. It's essential to recognize that while the output resembles a binary number, it's indeed a string.
To illustrate, consider the following:
bin(10)
This command translates the decimal number 10 into its binary counterpart.
'0b1010'
For a more nuanced understanding, let's input a negative integer, such as bin(-10).
bin(-10)
The output string will bear a negative sign preceding the "0b" prefix, encapsulating the binary representation of the negative integer.
'-0b1010'
It's worth reiterating that the bin() function's output is a string representation, not an actual binary number.
Should you intend to conduct mathematical operations on the result, a conversion back to an integer is necessary.
For instance:
a=bin(2)
b=bin(3)
Here, "a" is assigned the string '0b10', and "b" receives '0b11'. These strings correspond to the binary representations of the numbers 2 and 3.
Attempting to add a and b directly.
a+b
Python interprets this as string concatenation, resulting in:
'0b100b11'
To compute the sum of their binary values, a conversion to decimal integers is paramount.
a=int(a,2)
b=int(b,2)
Post-conversion, "a" holds the integer 2, and "b" contains 3.
Performing the addition:
a+b
Python now computes the sum of 2 and 3, yielding:
5