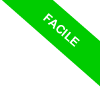
Python's eval() Function
The eval() function in Python is a powerful tool that evaluates an expression embedded within a string and returns its result.
eval(string)
The argument nestled within the parentheses is a string, which can encapsulate either a number or a mathematical-logical expression.
Once invoked, the function seamlessly performs the calculations and yields the corresponding numerical outcome.
A word of caution. The function eval() doesn't merely evaluate expressions, it has the capability to execute code. Given the potential security implications and vulnerabilities, it's prudent to employ `eval()` exclusively with strings of verified provenance. Exercise discretion when leveraging this function.
One common application of eval() is converting strings into numerical values.
Consider the string "-10" assigned to the variable 's':
s="-10"
Applying the eval() function:
eval(s)
This operation transforms the string into its numerical counterpart, -10.
-10
Exploring further, let's evaluate a string containing an arithmetic expression, such as "-10+7".
eval("-10+7")
Here, eval() processes the expression, culminating in a result of -3.
-3
Interestingly, `eval()` is versatile enough to interpret strings with embedded variables.
For instance, after assigning the integer 7 to the variable x:
x=7
Processing the string "-10+x" with eval()
eval("-10+x")
Yields a consistent output of -3.
-3
Venturing into more complex territory, consider the string "0b1010+0o14+0x1f", a composite of binary, octal, and hexadecimal values.
eval("0b1010+0o14+0x1f")
The function eval() adeptly converts these values to their decimal equivalents, aggregates them, and delivers a sum of 53.
53
If presented with a purely alphanumeric string devoid of computational elements, eval() simply returns the unaltered string. For instance:
eval(" 'Hello' ")
Hello
It's imperative to underscore that the eval() function, while potent, can execute Python code.
This capability can inadvertently introduce security vulnerabilities to your application.
For demonstration, processing the string:
eval("print('Hello')")
The `eval()` function dutifully executes the `print()` command, rendering "Hello" on the screen.
Hello
In summation, while `eval()` is undeniably powerful, it's judicious to deploy it sparingly, ensuring strings are sourced from trusted origins and approached with caution.
Avoid, at all costs, executing user-inputted strings.
For those seeking a safer alternative, consider the `ast.literal_eval()` function. It's designed to evaluate only literal expressions, such as tuples, strings, and numbers, without the risk of executing arbitrary code. Additionally, excessive reliance on `eval()` can inadvertently hamper your program's efficiency, given its interpretative nature with each invocation.