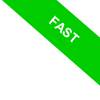
The int() Function in Python
In Python, when you're looking to transform a given value into an integer, the int() function is your go-to tool.
int(x)
In this context, `x` can either be a float or a string that holds numeric characters.
The outcome? An integer representation of that value.
But here's where it gets interesting. If you're dealing with a string that signifies an integer in a non-decimal base (like hexadecimal, octal, or binary), you'll need to bring in a second argument to specify the base.
int(x, base)
Let's break this down with an example.
Suppose you have the string "123" and you assign it to a variable, "x".
x = "123"
To morph this string into its numeric counterpart, you'd use int(x)
int(x)
And just like that, you get the integer 123.
123
But let's not stop there.
Consider you have a float, say 123.5, and you assign it to "x".
x = 123.5
Converting this float to an integer is straightforward
int(x)
The result? A neat 123.
123
Now, for those strings that represent numbers in bases other than 10, always remember to specify the base.
Take the string "A1" for instance.
x = "A1"
This isn't just any string. "A1" represents a number in hexadecimal, or base 16.
So, to get its integer form.
int(x,16)
Voilà! You're presented with the integer 161.
A word of caution: attempting to convert a non-numeric string, like "123go", will have Python raising its eyebrows—and a "ValueError" exception. Always ensure your input is valid to avoid any hiccups.