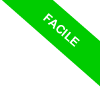
The oct() Function in Python
In Python, there exists a built-in function aptly named oct(). Its primary role is to convert integers into their corresponding octal representation, presented as a string.
oct(number)
In this context, "number" represents the integer you intend to convert.
Upon invoking this function, the returned string will bear the "0o" prefix, the standard notation for octal numbers within the Python environment.
This string denotes the number in base 8, employing digits ranging from 0 to 7. It's imperative to recognize that this output, while resembling a number, is indeed a string and thus not suitable for arithmetic operations.
For clarity, consider the following example.
Execute the command oct(10).
oct(10)
The function translates the integer 10 into the string '0o12', where 12 stands as the octal equivalent of 10.
'0o12'
Venturing further, let's evaluate a negative integer.
Input the command oct(-10).
oct(-10)
In this scenario, the function renders the decimal integer -10 as the string '-0o12'.
'-0o12'
Given that `oct()` produces strings, mathematical operations require an additional step. To perform arithmetic with the results, one must first revert these strings to numerical form. The subsequent example elucidates this process.
Suppose we define two variables, "a" and "b":
a=oct(2)
b=oct(3)
A direct summation of a+b results in a concatenated string.
a+b
'0o20o3'
To achieve an accurate summation, it's necessary to first transform the strings back into their integer form.
a = int(a, 8)
b = int(b, 8)
Upon executing a+b, the result is the aggregate of the two integers, yielding 5.
a+b
5
Through these illustrative examples, the nuances of the oct() function and its effective utilization within Python have been elucidated.