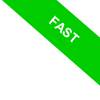
Python's type() Function
In the realm of Python, the type() function is a particularly useful tool, effectively acting as a mirror to the data type of an object.
type(x)
Imagine 'x' as the object you're interested in. You're curious about its type - and who can blame you?
And that's where type() jumps in. This function proudly presents the type of your object, it could be "int", "float", "string", among others.
Here's a key feature of Python that sets it apart: its dynamic type system. There's no need to declare a variable type when you bring it to life. Python's got your back; it automatically determines the data type based on the value you provide. If, for example, you define the variable:
year=2020
Python gives it the "int" treatment because 2020 is, of course, an integer. And the process is just as straightforward for other types.
Let's walk through a hands-on example.
Imagine defining a variable, then blessing it with the value "Hello World":
y = "Hello World"
Then, apply the type() function to reveal the data type of the object snugly assigned to variable "y":
print(type(x))
In this scenario, type() unveils the output <class 'string'>.
<class 'string'>
This implies that the variable "y" has been gifted with a string, essentially an alphanumeric value.
On the subject of Python's Data Types. Python comes equipped with a variety of built-in data types. These can be discerned using our handy type() function. A few examples include:
- int: tailor-made for integer numbers, e.g., 10, 2, 89.
- float: designed for numbers with a decimal part, e.g., 2.3, 3.14, 0.99.
- str: a perfect fit for text strings, e.g., "hello", "Python", "123".
- list: specifically for lists of objects, e.g., [1, 2, 3], ["a", "b", "c"].
- dict: ideally suited to dictionaries, collections of key-value pairs, e.g., {"name": "Mario", "age": 30}.
- bool: a binary choice of boolean values, namely True or False.
Expanding the type() Function to Custom Types
The usefulness of the type() function doesn't stop at Python's built-in data types. No, indeed, it extends its reach to custom data types that you can craft yourself.
Imagine, for example, defining the class Person:
- class Person:
- def __init__(self, name, age):
- self.name = name
- self.age = age
Then you conjure up an instance of the class in the variable "x"
x = Person("Mario", 30)
To check if the variable "x" is an instance of that class, you can once again call upon type()
print(type(x))
In this context, type() serves up the output <class '__main__.Person'>, affirming that variable "x" is indeed an instance of the Person class.
<class '__main__.Person'>
To sum things up, the type() function serves as a swift and reliable means to identify a variable's type, whether you're mingling with Python's built-in data types or custom ones of your own creation.
In the trenches of debugging a program, it proves itself to be an invaluable ally.