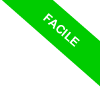
Python's hex() Function
Python's built-in hex() function offers a streamlined approach to converting integers into their hexadecimal counterparts.
hex(number)
Here, "number" denotes the integer you aim to convert.
What can you expect? A string prefixed with "0x", representing the number in hexadecimal.
This "0x" prefix is Python's standard notation for hexadecimal values. The hexadecimal system employs 16 distinct characters: the digits 0 through 9 and the letters 'a' through 'f'.
Let's delve into a practical example.
Execute the command hex(10) within Python's interactive shell.
hex(10)
In hexadecimal, the number 10 translates to the symbol "A".
Thus, executing the function yields the string '0xa'.
'0xa'
Now, consider a negative integer, such as hex(-10).
hex(-10)
Here, the negative sign precedes the "0x" prefix, resulting in:
'-0xa'
It's crucial to understand that the hex() function outputs a string, not an actual hexadecimal number.
Consequently, it's not suitable for direct mathematical operations.
Imagine attempting to sum two hexadecimal values:
a=hex(2)
b=hex(3)
At this juncture, "a" stores '0x2', and "b" contains '0x3'.
However, a simple a+b won't yield the anticipated sum.
a+b
In Python, when dealing with strings, the "+" operator concatenates rather than adds.
Thus, '0x2' and '0x3' merge to form:
'0x20x3'
To achieve the desired addition, first convert the hexadecimal strings to integers using the int() function.
Remember to specify 16 as the base.
a=int(a,16)
b=int(b,16)
Now, the sum of "a" and "b" provides the expected result: 2+3=5.
a+b
5
Once you've obtained the decimal result, you can effortlessly revert to the hexadecimal format using the hex() function.