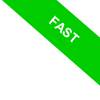
The "nan" Symbol in Python
In Python, "nan" or "NaN" is an acronym for "Not a Number". It's a special representation for an undefined or invalid numerical value.
Consider this: when you attempt to divide infinity by infinity:
x=float('infinity')
y=float('infinity')
print(x/y)
Python responds with the "nan" symbol. Why? Because the mathematical operation ∞/∞ doesn't have a defined outcome.
nan
This indicates that the result isn't a valid number It's either undefined or simply can't be represented.
Interestingly, any arithmetic involving "nan" and other numbers will invariably produce "nan
To assign this unique value to a variable, you can employ the float function:
x=float('nan')
For those seeking alternatives, the 'nan' value is accessible via the math library
import math
x = math.nan
Or, if you're working with the numpy library
import numpy as np
x = np.nan
Now, if you were to add x to 1, Python consistently returns "nan"
x+1
nan
Here's a curious aspect of 'nan': it's not considered a number in the traditional sense. In fact, it's not even equivalent to itself.
Testing its equality might surprise you:
x=float('nan')
print(x==float('nan'))
False
To effectively compare "nan", it's advisable to use the isnan() function from the math module:
import math
x=float('nan')
print(math.isnan(x))
True
For those leveraging the numpy module, a similar function exists:
import numpy as np
x=float('nan')
print(np.isnan(x))
True
To wrap things up, it's crucial to differentiate between NaN and None in Python.
While "nan" denotes an invalid or undefined numerical value, "None" represents a value's absence or a null state.