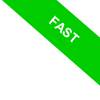
Python's Decimal Module
Python's Decimal module is a game-changer for those seeking precision beyond the standard float format. Not only does it offer a higher degree of accuracy, but it also supports an impressively broad range of values.
Included in Python's standard library, the Decimal module shines particularly in financial and scientific domains, where the exactness of calculations is non-negotiable.
Let's explore this with a practical example.
First, let's initialize the Decimal module:
import decimal
Curious about the maximum exponent value for representing a number? Let's check it out:
print(decimal.MAX_EMAX)
999999999999999999
This expansive range starkly contrasts with the limitations of floats.
But the real magic lies in its precision. Consider two floating-point numbers:
f1 = 0.1
f2 = 0.2
Combine them, and the result might surprise you:
float_sum = f1 + f2
print(float_sum)
Instead of a clean 0.3, you'll often get:
0.30000000000000004
This discrepancy stems from the inherent precision challenges with floating-point numbers.
Now, let's replicate this with the Decimal module. Define two numbers:
d1 = decimal.Decimal('0.1')
d2 = decimal.Decimal('0.2')
Sum them up:
decimal_sum = d1 + d2
print(decimal_sum)
Voilà! A perfect:
0.3
By leveraging the Decimal module, we bypass the typical precision pitfalls associated with floating-point numbers.
However, it's essential to understand the trade-offs. While operations with floats tend to be faster (thanks to hardware-level support in most CPUs), they often compromise on precision.
In summary, while floats might be the go-to for speed and general applications, decimals are the gold standard when precision is paramount, even if it demands a tad more computational time.