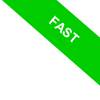
Python's is_integer() Method
In Python, the is_integer() method serves a specific purpose: it determines if a given floating-point number can be classified as an integer. Consider this syntax:
obj.is_integer()
Here, "obj" refers to an object of type float.
So, what does this method return? If the floating-point number is effectively an integer, it gives back a boolean value of True. Otherwise, you'll receive `False`.
To put it simply, `is_integer()` inspects a floating-point number to see if there's a fractional component. Take the number 5.0, for instance. While it's a float, it's also an integer because it lacks a decimal portion. But remember, this method is strictly for float objects. Should you apply it to an integer or a different data type altogether, you're inviting Python to raise an AttributeError.
Let's delve into a practical example.
Suppose you assign the number 5.3 to a variable named num.
num=5.3
Naturally, the datatype of num is float:
type(num)
<class 'float'>
Now, if you were to apply the is_integer() method to our variable.
num.is_integer()
The output will be False, simply because 5.3 isn't whole.
False
Consider another example for clarity.
Assign the number 5.0 to a variable, say num2
num2=5.0
Given that 5.0 is a float, the type of num2 remains float.
type(num2)
<class 'float'>
And on applying the is_integer() method
num2.is_integer()
The output is True, indicating that the number, while being a float, is effectively an integer.
True
This seemingly modest method boasts a spectrum of applications.
It can be instrumental when you need to verify user input as being either an integer or decimal. Moreover, during data evaluations, it's a swift way to check the integer status of values. For those dabbling in number theory or discrete mathematics, its utility cannot be overstated.
In conclusion, while the is_integer() method might appear rudimentary, it's an indispensable tool for developers, precluding the need to craft bespoke algorithms for integer checks.