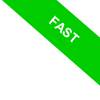
Python's del() Function
Let's talk about Python's impressive del() function, which is a powerful tool for managing data structures in Python. With it, you can easily remove an item from a list or dictionary using a simple yet effective syntax:
del(object[index])
Here, "object" refers to the name of your list or dictionary, and "index" is the position of the item within that structure.
However, the versatility of del doesn't stop there. You can employ it to delete variables and sets too.
del variable
One important thing to keep in mind is that the del statement isn't a method. This means it doesn't adhere to the dot syntax, making object.del() an invalid command.
Del is not tied to a specific object type, but it's a Python keyword with a wide reach. It has the ability to delete various types of objects, though it does have some limits. For example, it can't be used to remove an item from a tuple or string, as these data types are immutable in Python.
Applying del() to Lists
Python's del command shines when used to eliminate an item from a list based on its position, or index. Here's how:
Let's say we have a list:
list = [1, 2, 3, 4, 5]
You want to remove the third item. You'd do it this way: del list[2]
del list[2]
The key thing to remember here is that Python's indexing starts from 0, which means that list[2] actually refers to the third item in the list.
After you've made the change, you can see it in action using the print() command.
print(list)
You'll see that the third item, with index 2, has been efficiently removed.
The list now reads [1, 2, 4, 5].
[1, 2, 4, 5]
Note that it removes elements straight from the original list without making a copy.
If you need the original list to remain untouched, you should create a copy first or employ the list.remove(x) method. This method neatly removes the first list item with a value of x. Another option is list slicing list[start:stop] which carves out a new list with elements from start to stop, excluding the stop itself.
Furthermore, del() can be wielded to remove multiple items from a list simultaneously.
For example, create a list.
list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Then use the del() function to remove the items from the third to the fifth position:
del list[2:5]
In this example, the command del list[2:5] removes items from the third to the fifth position (indices 2, 3, and 4).
Now print the list.
print(list)
The list's content is now [1, 2, 6, 7, 8, 9, 10]
[1, 2, 6, 7, 8, 9, 10]
Applying del() to Dictionaries
The del() function also enables you to deftly remove a key and its associated value from a dictionary. Here's a quick example:
Consider a dictionary:
dictionary = {'one': 1, 'two': 2, 'three': 3}
Now, you decide to delete the key "two". You'd do this:
dictionary['two']
Once the modification is done, print the dictionary:
print(dictionary)
And there you have it, the key "two" and its associated value are no more.
{'one': 1, 'three': 3}
Applying del() to Sets
Interestingly, del() can also be put to work in erasing a whole set.
Suppose you have a set:
s = {1, 2, 3, 4, 5}
To delete the set, use this command: del s
del s
This command swiftly removes the entire set from memory.
Using del() with Variables
The usefulness of del extends to clearing a variable from memory.
What this means in practical terms is that once a variable is removed, your program will no longer recognize its name.
Let's walk through an example, create a variable:
x = 10
Try printing it.
print(x)
Python dutifully displays the value stored in the variable, which is 10 in this case.
10
To delete the variable from memory, use this command:
del x
If you now attempt to display the variable's content using the print() command, Python will raise an exception, because the variable simply doesn't exist anymore.