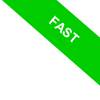
Combinations with Repetitions in Python
The combinations_with_replacement() function, a feature of Python's itertools module, is a powerful tool for generating all possible combinations of a given length from an iterable, such as a list, including repetitions.
combinations_with_replacement(iterable, r)
This function requires two arguments:
- iterable: The source iterable (like a list or string) from which the combinations are formed.
- r: The length of each combination, specified as a non-negative integer.
It yields every possible r-length combination from the iterable's elements, duplicates included.
Significantly, this function embraces element repetition within combinations. For example, with an iterable containing [A, B, C], combinations of two will not only include pairs of distinct elements like [A, B], [A, C], and [B, C], but also pairs of the same element, such as [A, A], [B, B], and [C, C]. To generate combinations without repetition, switch to the combinations() function from the same itertools module.
Before you can use combinations_with_replacement(), it must be imported from the itertools module.
from itertools import combinations_with_replacement
Let's dive into a practical example:
Consider a list named elements = [A, B, C], and your task is to find all two-element combinations, allowing for repetition.
- from itertools import combinations_with_replacement
- elements = ['A', 'B', 'C']
- combinations = list(combinations_with_replacement(elements, 2))
- print(combinations)
Executing this script will produce a list of every two-element combination, including those with repeated elements.
The output includes pairs like ('A', 'A'), ('B', 'B'), and ('C', 'C'), alongside combinations of distinct elements.
[('A', 'A'), ('A', 'B'), ('A', 'C'), ('B', 'B'), ('B', 'C'), ('C', 'C')]
It's important to note that in the context of combinations, the sequence of elements is irrelevant. Thus, the pair ('A', 'B') is identical to ('B', 'A'), and only the former appears in the results.
A word of caution for large datasets: The total number of combinations can escalate quickly, demanding substantial memory and processing time, which can affect overall efficiency. In such scenarios, it's advisable to use this function with care.
This itertools function is invaluable for developing statistical applications and solving complex problems involving combinations with repetition, all without the need for extensive coding.