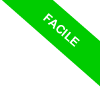
Python's takewhile Function
The takewhile() function is a powerful tool for creating iterators that retrieve elements from an iterable, such as a list, as long as a specific condition is satisfied.
takewhile(predicate, iterable)
This function requires two parameters: a function that sets the condition, and the iterable from which elements are to be extracted.
- predicate is the function that determines the condition for each element in the iterable.
- iterable refers to the data structure, like a list or tuple, from which elements are drawn.
It produces an iterator, yielding elements from the iterable as long as the specified function returns True.
This function proves invaluable when handling sequences or iterables, particularly when there's a need to select elements based on a defined criterion.
The takewhile() function is a component of the itertools module in Python.
Therefore, to utilize it, you must first import the itertools module or the takewhile function itself:
from itertools import takewhile
Consider the following practical application of takewhile().
Suppose you have a series of numbers and you wish to extract numbers sequentially until you encounter one that fails to meet a certain criteria.
Begin by defining a straightforward function, is_less_than_5(), to verify if an element is smaller than 5.
- def is_less_than_5(x):
- return x < 5
Create a list of numbers.
numbers = [1, 4, 6, 4, 1]
Now, employ takewhile to fetch numbers until a number not satisfying the "less than 5" condition is encountered.
result = takewhile(is_less_than_5, numbers)
In this instance, takewhile() persistently selects elements from the "numbers" list, submits them to the is_less_than_5() function, and appends them to the "result" iterator until it finds a number exceeding 4.
The process of extracting elements stops once the is_less_than_5() function yields False.
Proceed to display the final result.
print(list(result))
Now, let's print the final result.
print(list(result))
In this example, the output is [1, 4] because the subsequent number, 6, fails to satisfy the condition established by the is_less_than_5 function.
[1, 4]
It's important to note that takewhile() generates an iterator as its output. As a result, if you're aiming to acquire a list as the final output, it's necessary to transform the iterator into a list. This can be achieved using the list() function.