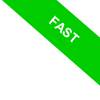
Python itertools' filterfalse() Function
The filterfalse() function, part of Python's itertools module, is a sophisticated tool for filtering iterable elements.
filterfalse(predicate, iterable)
This function requires two inputs: a predicate function and an iterable.
- The predicate function is applied to each item in the iterable, yielding a boolean value — True or False.
- An iterable refers to any collection that can be looped over, such as lists, tuples, sets, or any Python iterable object.
What makes filterfalse() stand out is its ability to generate an iterator with elements from the iterable that make the predicate function return False.
Before diving into filterfalse(), ensure you've imported the itertools module:
import itertools
Alternatively, import only the filterfalse function:
from itertools import filterfalse
Let's explore a real-world example.
In this script, we define an is_even() function to determine if a number is even or odd. We also have a list of numbers, aptly named "numbers".
- from itertools import filterfalse
- def is_even(n):
- return n % 2 == 0
- numbers = [1, 2, 3, 4, 5, 6]
- odd_numbers = filterfalse(is_even, numbers)
- print(list(odd_numbers))
filterfalse() methodically works through each number in the list, consulting is_even() for each. This function returns True for even numbers and False for odd ones.
Consequently, filterfalse() compiles an iterator exclusively with elements that elicited a False from is_even() — in essence, the odd numbers.
[1, 3, 5]
This technique effectively isolates all the odd numbers from your initial list.
Keep in mind that filterfalse() yields an iterator. To access the filtered values, iterate over it or convert it into a list using the list() function, as demonstrated above.
filterfalse() is also compatible with lambda functions.
For example, the following script achieves the same goal using a lambda function:
- numbers = [1, 2, 3, 4, 5, 6]
- odd_numbers = filterfalse(lambda x: x % 2 == 0, numbers)
- print(list(odd_numbers))
Here, filterfalse() picks out all numbers from the list that don't satisfy the lambda function's criteria, presenting them as the output.
The result? A list of the odd numbers from the original "numbers" list.
[1, 3, 5]
In summary, filterfalse() is an invaluable function for excluding elements from an iterable based on a defined condition. Its versatility makes it a go-to tool in various Python programming scenarios.