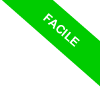
Python's dropwhile() Function
The dropwhile() function, a feature of Python's itertools module, provides an elegant way to filter sequence elements based on a given condition. It continues to omit elements as long as a specified condition remains true.
dropwhile(predicate, iterable)
This function requires two parameters:
- predicate: a function that defines the condition to be evaluated. It accepts a sequence element, returning either True or False. This can be a custom-defined function or a succinct lambda function.
- iterable: the sequence (like a list, string, or set) from which elements are drawn.
Elements from the iterable are excluded as long as the predicate function yields True. As soon as an element fails this condition, dropwhile() shifts its behavior, yielding all subsequent elements without further condition checks.
Importantly, dropwhile() does not alter the original sequence but creates a new sequence of elements, resulting in a new iterator.
Let's look at a practical example:
Begin by importing the dropwhile function from the itertools module:
from itertools import dropwhile
Create a number list:
numbers = [1, 3, 4, 7, 9, 1]
Imagine you wish to discard numbers less than 5. To achieve this, apply the dropwhile() function with a lambda function that returns True for numbers less than 5:
result = dropwhile(lambda x: x < 5, numbers)
The lambda function deems the first three elements (1, 3, 4) as True, but encounters a False with the number 7. Subsequently, all remaining numbers are included in the output.
Display the resulting sequence:
print(list(result))
The resulting sequence is [7, 9, 1], which is arrived at by omitting the initial elements (1, 3, and 4) that are less than 5.
[7,9,1]
Notice the presence of a value less than 5 in the output. This illustrates how dropwhile() ceases its filtering operation once a False condition is encountered.
Keep in mind that dropwhile() generates an iterator. To transform this into a list or another data format, utilize functions such as list(), set(), etc.
In conclusion, dropwhile() serves as a powerful tool for selectively filtering sequence elements, particularly useful when the goal is to ignore an initial subset of data.