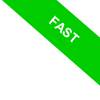
The islice() Function in Python
The islice() function, part of Python's itertools module, is an efficient way to extract a subset of elements from an iterable, such as a list or generator. This method is especially useful as it avoids the need to copy the entire iterable into memory.
itertools.islice(iterable, start, stop[, step])
Let's break down its arguments:
- iterable: The source from which elements are extracted. This can be any iterable, like a list, tuple, or generator.
- start: The index at which extraction begins, included in the results.
- stop: The index where extraction ends, not included in the results.
- step (optional): Determines the interval for extraction. For example, a step of 2 selects every second element, skipping the ones in between.
islice() returns an iterator that yields a specified slice of elements, based on your defined start, stop, and step indices.
Note: islice() outputs an iterator. To convert this to a list, use the list() function. It's also worth mentioning that islice() does not support negative indexing.
Here’s a step-by-step guide to using islice().
Firstly, import the function from the itertools module.
from itertools import islice
Next, create your iterable, like a list:
iterable = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Now let's use islice() to extract a range of elements, say from index 2 to 5:
sliced = islice(iterable, 2, 6)
The sliced elements are stored in the variable sliced.
To view these as a list, simply print:
print(list(sliced))
This will display the elements from index 2 to 5:
[2, 3, 4, 5]
Now, for another example:
Using islice(), let’s extract only the first 5 elements of the iterable:
sliced = islice(iterable, 5)
print(list(sliced))
This will start at the beginning and select the first five elements:
[0, 1, 2, 3, 4]
Exploring the Step Parameter
islice() also allows you to incorporate a "step", akin to list slicing. Let's see an example:
sliced = islice(iterable, 2, 9, 2)
print(list(sliced))
This results in a list containing every second element from index 2 up to 8:
[2, 4, 6, 8]
Working with Generators
islice() is particularly useful when dealing with generators, as traditional slicing methods are not applicable.
- def gen_numbers():
- n = 0
- while True:
- yield n
- n += 1
- iterable = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
- gen = gen_numbers()
- sliced = islice(gen, 5, 10)
- print(list(sliced))
The output is a list with the elements from 5 to 9:
[5, 6, 7, 8, 9]
These examples illustrate the basics of using islice() in Python, making it an excellent tool for managing large datasets or working with generative sequences without the need for loading the entire sequence into memory.