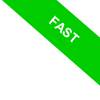
The itertools.count() Class in Python
In Python, the itertools.count
class is your go-to for creating an endless counter, churning out a continuous sequence of numbers.
count(start=0, step=1)
Kick things off with an initial value (start
) and bump it up by a step increment (step
) with each cycle iteration.
It's a real game-changer for crafting iterative loops.
Tucked inside the itertools module, you need to bring it into play by importing it from itertools
. The count
class is a common pick for scenarios that call for an unbounded stream of values.
Here's a hands-on example.
import itertools
counter = itertools.count(start=10, step=2)
This script fires up an iterator that spits out an endless string of numbers beginning at 10, climbing by 2 each time.
So, it'll reel off numbers like 10, 12, 14, 16, and so on, into infinity.
Take it for a spin with the next()
function.
print(next(counter))
The iterator dishes out 10, the starting point of our never-ending sequence.
10
Now, let's hit it again with next()
.
print(next(counter))
The iterator hands us 12, thanks to our step-up of 2.
12
Bear in mind, itertools.count
whips up an infinite iterator, so it's wise to pair it with some stop conditions in your loops, dodging the bullet of an eternal loop.
One of the slickest moves with count
is pairing it with zip
to tack a counter onto a list or to swap out the classic indexed for
loop when needed:
Check out this snippet:
- for index, value in zip(itertools.count(1), ['a', 'b', 'c']):
- print(f"{index}: {value}")
This bit of code employs a for
loop to march through two sequences in lockstep: an infinite counter starting at 1 and a character list ['a', 'b', 'c']
.
Each pass prints out an index-value pair.
1: a
2: b
3: c
If iterators and iterable objects are your bread and butter in Python, the itertools.count
class is something you ought to be familiar with.