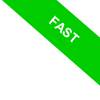
Python's itertools.repeat() Function
Within the itertools module of Python lies the repeat() function—a nifty tool for replicating a single value as many times as needed. Let's dive into its syntax and utility:
repeat(object[, times])
The function is straightforward with two parameters:
- object is the item you want to duplicate. It's the only required parameter.
- times is an optional count of how many times you want the `object` to be repeated. Without specifying `times`, `repeat` will endlessly churn out duplicates, so tread lightly if you choose to omit this argument.
What you get in return is a stream of the object, replicated over and over.
This utility shines when you're looking to lock a value across several iterations or when crafting a data sequence with uniform elements.
The repeat() function is a component of the itertools module.
Before putting it to work, you'll need to bring it into your Python environment with the following import statement:
from itertools import repeat
With the import out of the way, repeat() is at your disposal for the duration of your Python session, no re-import necessary.
Let's put it into action. Imagine you want to generate a sequence where 'X' appears 5 times:
- for i in repeat('X', 5):
- print(i)
This for loop will output 'X' five times, echoing it to your terminal like so:
X
X
X
X
X
But repeat() isn't just a one-trick pony—it's equally adept in list comprehensions and function maps.
Take this example, where we add three to each number in a list using the map() function:
numbers = [1, 2, 3, 4]
sums = map(lambda x, y: x + y, numbers, repeat(3))
print(list(sums))
This code effortlessly produces [4, 5, 6, 7], incrementing each number in the original list by three.
[4, 5, 6, 7]
Repeat() also excels when paired with functions that handle multiple iterable objects.
Consider a scenario where you have a list of numbers that you'd like to exponentiate. Say you want to square each number:
- from itertools import repeat
- # List of numbers to be squared
- numbers = [1, 2, 3, 4, 5]
- # The exponent is a fixed value
- exponent = 2
- # Applying `pow` to each list element using `map`
- # The `repeat(exponent)` ensures each `pow` call receives the value 2
- powers = map(pow, numbers, repeat(exponent))
- print(list(powers))
The result is a new list: [1, 4, 9, 16, 25], each number a square of the original.
[1, 4, 9, 16, 25]
In this instance, repeat() serves as an infinite supplier of the exponent value, seamlessly integrating with each iteration of the map cycle.
With a minimalistic approach, you can conjure up repetitive datasets and tackle complex iterable operations with finesse.
Without repeat, you'd be stuck manually crafting an iterable filled with duplicates or wrestling with a more intricate list comprehension or for loop to achieve the same end.
In sum, repeat() is a prime example of how a deceptively simple function can be a powerhouse in the right hands.