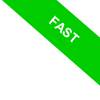
The itertools.cycle() Function in Python
Python's itertools.cycle() crafts an iterator that perpetually loops through the elements of the given input.
itertools.cycle(iterable)
Imagine an endless merry-go-round of elements from an iterable like a list or a string—that's what cycle() creates.
It cycles through each element and, upon reaching the end, seamlessly circles back to the start.
This function shines when you're aiming for repeated iterations over a constant set of data. It's ideal for emulating patterns that naturally loop, such as the stages of traffic lights, or when generating a consistent stream of test data.
Let's walk through a straightforward example:
- import itertools
- counter = itertools.cycle('ABCD')
- # Let's take a string as an example
- for i, letter in enumerate(counter):
- if i > 7:
- break
- print(i, letter)
This snippet will output the sequence of letters from 'ABCD':
0 A
1 B
2 C
3 D
4 A
5 B
6 C
7 D
Notice how after 'D', it picks right back up at 'A'? That's cycle() in action.
The loop halts when i > 7, providing a necessary escape from the otherwise endless loop.
A Word of Caution. Exercise discretion with itertools.cycle()—it's an infinite iterator by nature. Without a well-placed break or another form of a stopping condition, you might find yourself in an eternal loop.
To wrap it up, itertools.cycle() is a powerful ally for tasks that demand an unbroken, repetitive sequence. It's simplicity and efficiency in one package, but like any tool, it's most effective when used with intention and care.