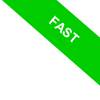
Python's itertools Module
Diving into Python, one quickly discovers the power and elegance of the itertools
module. Designed specifically for crafting and manipulating iterators, this module offers a robust set of tools that are both memory-efficient and adept at handling sequences and iterables.
So, what exactly are iterators? In Python, iterators are sophisticated objects that come equipped with the __iter__()
and __next__()
methods. While they share some similarities with lists or tuples, iterators have a distinct advantage: they generate values on-the-fly, ensuring minimal memory footprint.
Here are some functions you can find in the itertools module:
Infinite Iterators
The name is a giveaway. These functions churn out an unending sequence of values. They're invaluable when a continuous stream of values is required.
However, a word of caution: their endless nature demands careful handling to avoid unintentional infinite loops. Always ensure an exit strategy, such as a break
statement within a loop, to keep things under control.
Count
Imagine a function that starts counting from a given point and just... keeps going. That's count
for you. It begins at a specified start
value and increments by a defined step
.
count(start=0, step=1)
For instance, this script outputs a sequence of numbers:
- from itertools import count
- for i in count(5, 3):
- if i > 20:
- break
- print(i)
This snippet, for instance, rolls out numbers from 5, increasing by 3 each time, and halts once it crosses 20.
5
8
11
14
17
20
cycle
This function takes an iterable as its argument and repeats its elements indefinitely in a continuous loop.
cycle(iterable)
Here's a practical example:
- from itertools import cycle
- counter = 0
- for item in cycle('ABCD'):
- if counter > 7:
- break
- print(item)
- counter += 1
The script outputs the following sequence of letters:
A
B
C
D
A
B
C
D
repeat
Sometimes, simplicity is key. Repeat
does one thing: it echoes a given element, either endlessly or a set number of times.
repeat(element, times=None):
For example:
- from itertools import repeat
- for item in repeat('X', 4):
- print(item)
The output is:
X
X
X
X
While these functions are straightforward, they become especially powerful when combined with other Python functions and constructs.
Combinatorics
For those with a penchant for mathematical combinations and permutations, itertools
is a treasure trove. Functions like product
, permutations
, and combinations
allow for intricate sequence manipulations, making them indispensable for combinatorial tasks.
product
This function returns the cartesian product of the provided iterables. Using the repeat parameter, you can specify how many times you want to replicate the iterable.
product(*iterables, repeat=1)
For instance:
from itertools import product
product('AB', repeat=2)
This function outputs:
('A', 'A'), ('A', 'B'), ('B', 'A'), ('B', 'B')
permutations
This function yields all possible permutations of length r from an iterable. If r isn't specified, it assumes the iterable's full length.
permutations(iterable, r=None)
For example:
from itertools import permutations
permutations('ABC', 2)
This function outputs:
('A', 'B'), ('A', 'C'), ('B', 'A'), ('B', 'C'), ('C', 'A'), ('C', 'B')
combinations
This function produces unique combinations of length r from the iterable. Unlike permutations, the order of elements doesn't matter here.
combinations(iterable, r)
For instance:
from itertools import combinations
combinations('ABC', 2)
This function outputs:
('A', 'B'), ('A', 'C'), ('B', 'C')
combinations_with_replacement
This function yields all possible combinations with repetition.
combinations_with_replacement(iterable, r)
For example:
from itertools import combinations_with_replacement
combinations_with_replacement('ABC', 2)
This function outputs:
('A', 'A'), ('A', 'B'), ('A', 'C'), ('B', 'B'), ('B', 'C'), ('C', 'C')
Filtering
Beyond crafting sequences, itertools
also excels at refining them. With functions like filterfalse
, takewhile
, and dropwhile
, you can sieve through iterables based on specific conditions, ensuring only the desired elements come through.
filterfalse
This function does the exact opposite of the built-in filter()
function. Instead of returning iterable elements for which the predicate function returns True, filterfalse
yields elements for which the predicate returns False.
filterfalse(predicate, iterable)
For instance, in this script, the condition is True for even numbers:
from itertools import filterfalse
result = filterfalse(lambda x: x%2 == 0, [1, 2, 3, 4, 5])
print(list(result))
Thus, the function outputs all elements that don't meet the selection criteria, i.e., odd numbers:
[1, 3, 5]
takewhile
This function yields elements from the iterable as long as the predicate returns True. Once the predicate returns False, the function stops and doesn't process further elements, even if subsequent elements in the iterable meet the condition.
takewhile(predicate, iterable):
For example:
from itertools import takewhile
result = takewhile(lambda x: x < 5, [1, 2, 3, 4, 5, 4, 3, 2, 1])
print(list(result))
The script selects all numbers less than 5 until it encounters the number 5.
[1, 2, 3, 4]
dropwhile
This function does the opposite of takewhile()
. It skips over iterable elements as long as the predicate returns True. Once the predicate returns False, the function starts yielding all subsequent elements, regardless of whether they meet the condition or not.
dropwhile(predicate, iterable)
For instance:
from itertools import dropwhile
result = dropwhile(lambda x: x < 5, [1, 2, 3, 4, 5, 4, 3, 2, 1])
print(list(result))
It outputs all numbers after encountering the first element (5) that doesn't meet the x<5 criterion.
[5, 4, 3, 2, 1]
islice
Although not strictly a filtering function, islice()
allows you to select a sub-sequence from an iterable, similar to list slicing notation. It can be used to fetch a specific range of elements from an iterable.
islice(iterable, start, stop[, step])
For instance:
from itertools import islice
result = islice([1, 2, 3, 4, 5], 1, 4)
print(list(result))
This selects elements from the list starting from the second position up to (but not including) the fifth position.
[2, 3, 4]
These are just a few of the myriad functionalities offered by itertools.