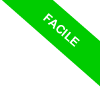
The chain Function in Python
The chain() function in Python elegantly enables iteration over several iterable sequences as if they were one cohesive sequence, eliminating the need for explicit joining.
itertools.chain(*iterables)
'*iterables' can be any collection of iterables, such as lists, tuples, or sets.
This function returns an iterator that seamlessly transitions between each iterable, yielding elements in sequence.
Significantly, 'chain' doesn't amalgamate elements into a new list; it sequentially iterates over each given sequence. This is particularly advantageous for large iterables, as it bypasses the extra memory and processing costs of creating new lists or tuples.
The 'chain()' function is a component of the itertools module.
To utilize this function, begin by importing itertools or the function directly.
from itertools import chain
'chain' can be applied to merge various iterables (like lists, tuples, sets, etc.) into one streamlined iterable.
Consider an example with three lists:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
Employing the chain() function, you can iterate across all these iterables in one go.
- for number in chain(list1, list2, list3):
- print(number)
This loop will output numbers from 1 to 9 in a unified sequence.
1
2
3
4
5
6
7
8
9
Chain is particularly useful for scenarios requiring the manipulation of multiple sequences as a single entity, contributing to cleaner and more efficient code.
If you're dealing with a list of iterables and aim to concatenate them, chain.from_iterable is an excellent choice.
- from itertools import chain
- list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
- for number in chain.from_iterable(list_of_lists):
- print(number)
This approach yields a concatenated sequence of all elements in the lists.
1
2
3
4
5
6
7
8
9
This method is invaluable when the number of iterables to be combined is not predetermined, offering flexibility and efficiency in handling such scenarios.