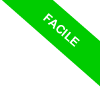
The itertools.permutations() in Python
The itertools.permutations function is Python's approach to calculating permutations of elements from any iterable.
itertools.permutations(iterable, r=None)
It accepts two parameters:
- iterable
This is the collection from which you want to generate permutations, be it a list, string, set, or another iterable structure. - r (optional)
An optional argument that sets the number of elements in each permutation. Supplying an integer for r results in permutations of that length. If you skip this argument or passNone
, the function defaults to permutations that include all elements of the iterable.
The function yields an iterator filled with tuples, each of length r, representing the permutations.
What exactly are permutations? In the realms of statistics and combinatorics, permutations are the various arrangements of a set of items where the sequence is crucial. They're essential for figuring out the number of distinct ways to order a set of items. Take three books, A, B, and C, for example. They can be arranged on a shelf in six different ways: ABC, ACB, BAC, BCA, CAB, and CBA.
Keep in mind, this function is a feature of the itertools module.
To put permutations into action, you need to import itertools at the beginning of your Python script or interactive session.
import itertools
Let's dive into a real-world example:
Start by defining a list with three elements.
A = [1, 2, 3]
Next, invoke the permutations() function to work its magic on the list.
B = itertools.permutations(A)
Here, permutations are calculated for the full length of the input iterable, which means sets of three for our list, resulting in an iterator of tuples that's stored in variable B.
To see the permutations, loop through B with a for loop and print each one.
- for p in B:
- print(p)
The output lists every possible ordering of the elements from list A.
(1, 2, 3)
(1, 3, 2)
(2, 1, 3)
(2, 3, 1)
(3, 1, 2)
(3, 2, 1)
To tailor the permutations to a specific length, simply specify your desired number of elements in the second parameter of the function.
For instance, consider this script:
- import itertools
- A = [1, 2, 3]
- B = itertools.permutations(A, 2)
- for p in B:
- print(p)
In this scenario, the function is generating permutations of the elements from list A in pairs.
Each tuple is a unique combination of two elements from A, showcasing the permutations of length r=2.
(1, 2)
(1, 3)
(2, 1)
(2, 3)
(3, 1)
(3, 2)
With this approach, you can effortlessly calculate permutations for any iterable object in Python.