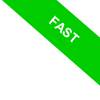
Python's combinations() Function
Today, we'll delve into the mechanics of calculating combinations with Python's itertools.combinations() function—a staple in the itertools module.
itertools.combinations(iterable, r)
At its core, the combinations function is straightforward, requiring two inputs: an iterable for sourcing elements and an integer to set the combination length. Here's how you break it down:
- iterable - the source iterable, like a list or string, whose elements are to be combined.
- r - the number of elements in each combination.
What this function does is simple yet powerful: it churns out every possible combination of elements from your iterable in groups of the size you've chosen.
Each combination is neatly packaged into a tuple, maintaining the original sequence of elements.
Combinations Explained: In the realm of statistics, 'combinations' describe the various ways you can select a number of items from a larger set where their order is irrelevant. Take three fruits, for example—an apple, a pear, and an orange. If you're curious about the different ways to pick two out of the three, you're looking at three combinations: apple with pear, apple with orange, and pear with orange.
Now, let's walk through its functionality and explore some hands-on examples.
Before you start, it's essential to import the itertools module to access the combinations function.
import itertools
Alternatively, you can import just the combinations function directly from itertools.
from itertools import combinations
Consider a scenario where you have a list of letters and your goal is to uncover all the 2-letter combinations possible.
- from itertools import combinations
- letters = ['a', 'b', 'c']
- pair_combinations = list(combinations(letters, 2))
- print(pair_combinations)
The combinations() function does the heavy lifting, presenting you with the potential combinations.
[('a', 'b'), ('a', 'c'), ('b', 'c')]
Notice how each tuple is a unique combination without repeats, and the order of selection is not factored in—('a', 'b') is treated the same as ('b', 'a').
Contrary to permutations, where the sequence matters, combinations focus on the selection of elements without regard to their arrangement. This also means no element is repeated within a combination.
The itertools.combinations function is an efficient and user-friendly tool for generating all possible combinations from a dataset. But a word to the wise: when dealing with large datasets and substantial combination lengths, the number of combinations can skyrocket, leading to increased memory and processing demands. Exercise caution under these conditions.