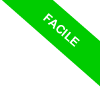
How to concatenate strings in python
In this lesson I'll explain how to do string concatenation in the Python language.
What is a string? A string is a sequence of alphanumeric characters (letters, numbers and symbols). For example "Python Tutorial" is a string.
In the python language to concatenate and join two or more strings you have to use the addition operator +
stringa1+stringa2
If the operands are alphanumeric values (eg strings or alphanumeric variables) the + operator joins the two strings, transforming them into a single string.
I'll give you some practical examples
Type and run this code
print("hello"+"world")
This script concatenates two strings and displays the result on the screen
helloworld
Example 2
Now write this other example code
Assign alphanumeric values to two variables (a, b).
Then it concatenates the two variables into a third variable (c).
a="Python"
b="Tutorial"
c=a+b
print(c)
The output on the screen is as follows:
PythonTutorial
Example 3
This script combines constants and alphanumeric variables with each other.
a="Python"
b="Tutorial"
print(a+" "+b)
The output on the screen is the union of the three strings
Python Tutorial
In the latter case there is a space between the values of the two variables, because in the concatenation there is also the alphanumeric constant.