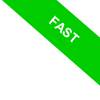
List Concatenation in Python
Python offers a wide range of solutions for list concatenation. Let's explore a few effective strategies to merge lists seamlessly.
Firstly, let's kick off by setting up two simple lists:
list1 = [1, 2, 3]
list2 = [4, 5, 6]
One of the most straightforward techniques to fuse these two lists into a new one is by employing the "+" operator:
list3 = list1 + list2
To visualize the resulting list, you can display list3 using print() function.
print(list3)
The output of this operation presents a new list housing elements from both list1 and list2, looking like this.
[1, 2, 3, 4, 5, 6]
Another viable alternative for concatenating lists is the extend() method.
list1.extend(list2)
Note that this approach directly modifies the original list, in this context, "list1".
To examine the updated list1:
print(list1)
This shows how list2's elements have seamlessly merged into list1, effectively extending it.
[1, 2, 3, 4, 5, 6]
Exploring Further Concatenation Techniques
If you're in a scenario where you're looking to iterate over a list and add elements to another list, the list.append() function is a suitable candidate for such a task.
Let's form two lists.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
Then, traverse list2, appending each of its elements to list1:
- for elemento in list2:
- list1.append(elemento)
Just like the extend() method, this technique alters the original list.
Now, list1 would be:
[1, 2, 3, 4, 5, 6]
Suppose you have a multitude of lists to concatenate, the itertools.chain() method can handle this efficiently.
Create three lists.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
Subsequently, bring in the itertools.chain method.
from itertools import chain
To merge all three lists into a single one.
list4= list(chain(list1, list2, list3))
To get a look at the result, print out the newly formed list.
print(lista4)
The output will showcase a single list incorporating elements from all three lists:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
That's it! With these methods at your disposal, you're now equipped to concatenate multiple lists efficiently, even with a single command.