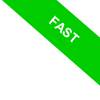
The Python join() Method
Ever found yourself needing to combine several strings in Python? Python's join() method is here to help. This useful function enables you to merge a list of strings into one, using a specific delimiter character that you choose.
delimiter.join(list_of_strings)
There are just two things you need to get started:
- The delimiter. This is the character or sequence of characters you want to use to separate the strings. It could be anything you like.
- The list of strings. This is the list of strings you want to merge. You just place it inside the parentheses.
Once you have these, the join() method merges the strings and gives back a single string.
It's important to note that the delimiter is a must. This is because it's the String object that you're applying the join() method to. However, if you want to join strings without using a delimiter, you can simply use an empty string "" as the delimiter. I'll go over how to do this shortly.
Let's go through an example.
First, create a list of strings.
>>> list_of_strings = ["Hello", "world", "how", "are", "you?"]
Next, choose a delimiter. A simple space " " is a common choice.
>>> delimiter = " "
Now, apply the join() method to the delimiter, adding your list of strings as an argument.
>>> delimiter.join(list_of_strings)
The result is a single string.
Hello world how are you?
This string consists of all the strings from the list, each separated by a space.
Remember, you can use any character or sequence of characters as the delimiter. For example, you can try using a string with two dashes "--"
>>> "--".join(list_of_strings)
Now, the join() method combines the strings, placing two dashes between each string.
Hello--world--how--are--you?
What if you want to join strings without a delimiter?
That's easy. Just apply the join() method to an empty string.
>>> "".join(list_of_strings)
This gives you a single string where all the elements from the list are combined together without any separation.
Helloworldhowareyou?
And that's a quick rundown on how to use Python's join() method. Have fun joining your strings together!